Python's OOP Wonderland: Classes and Objects Explored
Explore Python's core concepts: unravel the magic of classes and objects. Enhance your coding skills with our comprehensive guide to object-oriented programming.
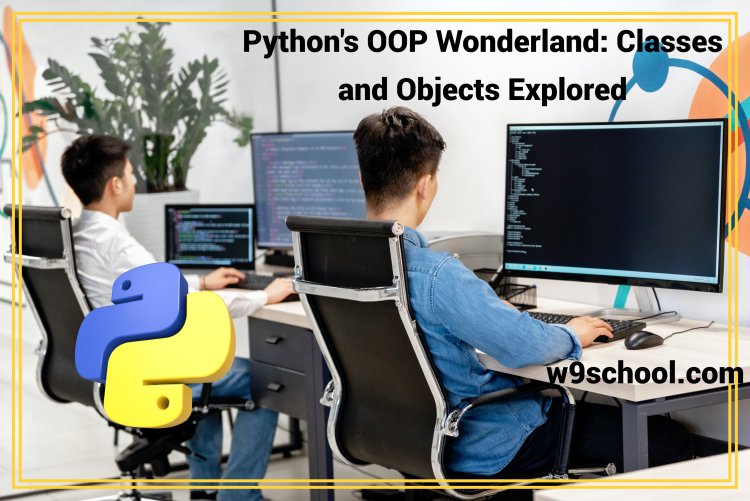
Python Classes and Objects |
Classes/Objects Python is an object-oriented programming language. Nearly everything that happens in Python has an element with attributes and functions. Objects and classes form the backbone of any Python program, without which programs would not be able to interpret user commands and function correctly. A deep knowledge of how Python classes and objects interact is vital for becoming a productive Python programmer. Python is an object-oriented programming language, so everything is treated as an object, making it easier for developers to write reusable and more organized code instead of opting for traditional procedural approaches. A class acts like a blueprint or template for multiple objects with similar attributes to be created at once. Python classes are user-defined data structures with attributes and methods created via calls to their methods. An instance can then be created by calling those methods, with each instance having its own set of attributes with unique values for those attributes that reflect an object's properties; additionally, each instance also comes equipped with its own methods that can help alter or modify this state of the object. Python allows you to create classes by including the class keyword followed by its name in your scripts. The name will serve as an identifier within your code; additionally, class definitions can include docstrings that outline its purpose and usage; this practice helps improve code clarity and readability.
One class can be derived from another by listing its parent class name in parentheses after the class name. This allows you to share attributes and methods from both classes between themselves or define new class attributes and methods to enhance existing data types (for instance, if an abstract data type is expected in code, you could create an abstract class that emulates its methods so as to avoid duplicating code). A class is more than its name: it also provides its own private and public methods, accessed using either class-level methods with self, which refer to instances of the class, or instance methods with an extra parameter called __self__ that work directly on individual objects to access or modify their state. Instance methods use individual instances as targets, while class methods operate across them all at the same time, allowing access to and manipulation of attribute values of objects within instances of that class. As part of your class development process, when writing classes, it's possible to add methods that return or set the values of attributes at runtime. Each instance of a class may have unique values for attributes; the class-level method allows you to manipulate these values to alter overall behavior without impacting all instances; this feature saves both time and effort when changing states without having to alter all instances at once. |
Creating A Class |
A Class can be described as an object constructor or "blueprint" for creating objects. In order to create classes make use of the term Example Create a class called MyClass and the property x:
|
Create Object |
We can now use the class called MyClass in order to make objects. Example Create an object p1 and output the value of the x:
|
The __init__() Function |
The above examples are objects and classes in their most basic form and they aren't really relevant in real-world applications. To grasp the significance of classes, we must be aware of the built-in init() functions. Every class has an init function, referred to as"__init__"() which is executed whenever the class is initiated. Make use of the function __init__() function for assigning values to property objects and other functions required while the object is being constructed: Example Create a class titled Person using the function __init__() function for assigning values to names and ages:
|
Notice: The |
The __str__() Function |
The function __str__() function determines the information that is returned when a class object is represented by strings. If the function __str__() operation is not defined the representation in string form of the object will be returned: Example The representation of a string object, minus the ______() functions:
Example The representation of a string object that uses the function __str__() method:
|
Object Methods |
Methods in objects can also be contained. Methods within objects refer to functions and are part of the object. Let's design an algorithm within the Person class: Example Create a function that prints an invitation, then execute it on the p1 object:
|
NOTE: The |
The self Parameter |
Self parameter is a reference to the current instance of class. It doesn't have to be identified as Example Make use of the terms MysillyObject or abc instead of self:
|
Modify Object Properties |
You can modify the properties of objects in this way: Example Set the age of p1 to 40:
|
Delete Object Properties |
You can remove properties from objects making use of del keyword: ExampleEliminate the age property of the object p1:
|
Delete Objects |
You can erase objects using Example Remove the object p1:
|
The pass statement |
Example
|
For more informative blogs, Please visit Home
What's Your Reaction?
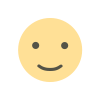
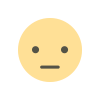
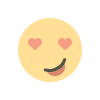
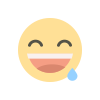
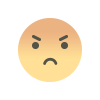
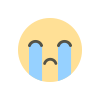
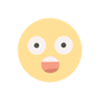