Python Lambda Functions: Unleashing Concise Coding Power
Dive into the world of Python Lambda functions! Explore concise coding techniques, streamline your code, and master the art of efficient programming effortlessly.
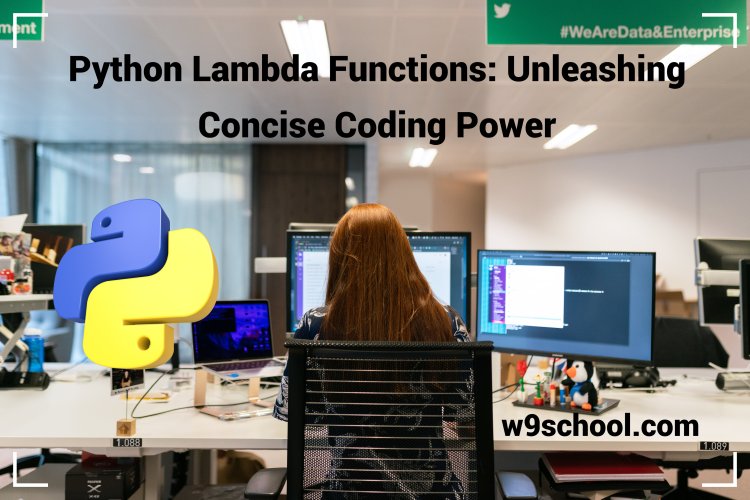
Python Lambda |
What is a Python Lambda?Python lambdas provide an efficient syntax for functions that return only one expression and are commonly used to implement functional programming techniques. Lambdas can also be found in user interface frameworks to map events to actions. While these functions may seem useful in all situations, it's essential to know when using them is suitable. FunctionsLambda functions are anonymous functions that do not have a defined identifier associated with them, similar to regular functions but can only perform one expression within their body. They cannot include return statements and should always be invoked immediately otherwise, they will raise a SyntaxError exception. Lambdas can be very useful when passing functions as arguments to other functions. For instance, popular functions like map() take an anonymous lambda expression and list as parameters before creating a new list based on the logic provided to it. Utilizing lambdas allows you to write code that is more concise and readable; however, their use is less efficient than using def statements, since no useful help or tracebacks are provided by using lambdas this way. However, lambdas remain an excellent solution for creating small functions. VariablesPython is an adaptable coding language with numerous built-in functions to assist with data manipulation, such as its lambda function that allows anonymous functions to be passed as arguments in lines of code - this feature is particularly helpful when working with user interface frameworks. However, lambda functions are limited by the variables available to them. While some languages offer workarounds for this issue, these solutions tend to be lengthy and inefficient. A general-purpose method for simulating variable assignment in lambdas would revolutionize Python golfing. Lambda functions can also directly pass their argument values, unlike def functions, which require users to list all arguments within parentheses. Furthermore, lambda functions must have all argument values available at the time of definition; otherwise they cannot be assigned later as variables. See this example using a lambda function that computes the square of two arguments as an example of this feature. ExpressionsLambda functions are part of functional programming styles which emphasize readability and avoid changing mutable data. Python offers lambda functions with any number of arguments they wish; they must only perform one expression as part of closure and return an integer value as their result. Lambda functions differ from regular functions in that they do not need to be enclosed in parentheses; rather, Python interpreter treats them just like any other function and generates their equivalent bytecode. Unfortunately this workaround may make reading your code cumbersome and inaccessible. Lambda functions can be used with higher-order functions like filter(), map() and reduce() to create iterable functions. They can also be combined together to form iterable functions; it is important to remember that lambda functions can only have one expression without returning a value; this differs from normal Python functions defined using def keyword which always return something tangible as their output value. StatementsThough lambda functions can cause some controversy within Python circles, the language fully supports and makes use of them. A lambda function may be assigned directly to variables without needing any return statement or return statement at all - making them highly convenient. Lambdas may accept multiple arguments but can only execute one expression at a time, so it is wise to carefully consider other possible solutions before turning to lambdas for your coding problem. For instance, if the code requires performing complex if-elif-else cycles then user-defined functions would likely provide better solutions than lambdas. Python lambdas are often employed in functional programming styles that use functions as arguments; map() and filter() are two popular examples that utilize lambdas for implementation in Python. To verify if these functions are functioning as expected, the dis keyword allows you to display a readable version of their Python bytecode and inspect its instructions. |
Creating A Python Lambda Fuunction |
Lambda functions are small, anonymous function. Lambda functions can accept an array of parameters but it is limited to one expression. Syntax
The expression is executed, and its result returned: Example Add 10 arguments to
Lambda functions can be triggered by any of the following arguments: Example Multiply argument
Example Summarize the argument
|
Why Use Lambda Functions? |
The potential of lambda is best demonstrated when you apply them as an unidentified function within another function. Let's say you have a definition that accepts one argument. The argument is multiplied by an unspecified number:
Make use of the function definition above to create an algorithm that will always double the amount you enter: Example
You can also use the same definition of function to create a function which always is triple the amount you input in: Example
Example You can also use an identical function description in order to perform both functions, using one program.
|
Use lambda functions if an anonymous function is needed for a brief period of time. |
For more informative blogs, Please visit Home
What's Your Reaction?
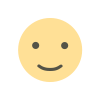
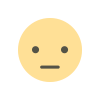
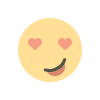
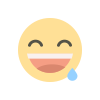
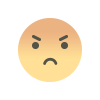
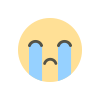
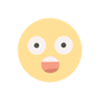