Python Operators Simplified: A Beginner's Guide to Mastery - w9school
Python operators are symbols and keywords used to perform operations on variables and values, making it a versatile language for computations.
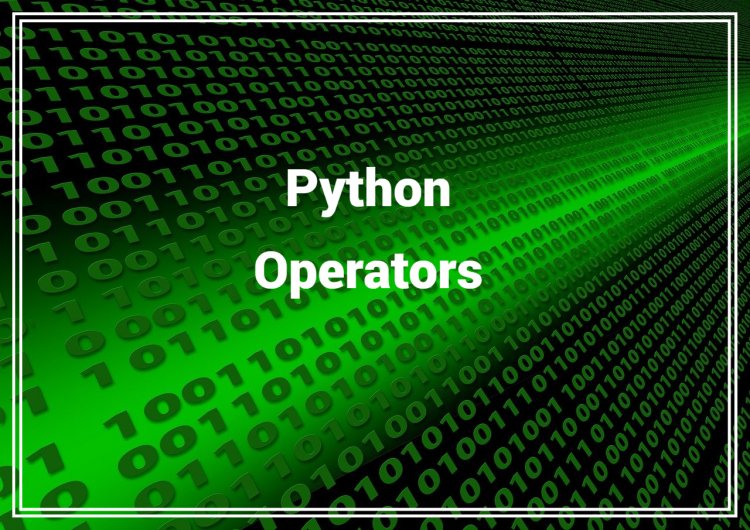
Python Operators |
Operators are used to perform operations on variables and values. In Python, operators are special symbols or characters that perform operations on operands (values or variables). Python provides a variety of operators to carry out different types of operations, such as arithmetic, comparison, logical, assignment, bitwise, and more. Let's explore the different categories of operators in Python: 1. Python Arithmetic OperatorsArithmetic operators are used with numeric values to perform common mathematical operations: - Addition: `+`
2.Python Comparison OperatorsComparison operators are used to compare two values: - Equal to: `==`
3. Python Logical OperatorsLogical operators are used to combine conditional statements: - Logical AND: `and` (returns True if both operands are True)
4.Python Assignment OperatorsAssignment operators are used to assign values to variables: - Assignment: `=` (assigns a value to a variable)
5. Python Bitwise OperatorsBitwise operators are used to compare (binary) numbers: - Bitwise AND: `&` (performs a bitwise AND operation)
6. Python Membership operatorsIn Python, membership operators are used to test whether a value is a member of a sequence or collection, such as a string, list, tuple, or set. These operators return a Boolean value of either True or False. Python provides two membership operators: 1. `in` operator: It checks if a value exists in a given sequence or collection. If the value is found, the operator returns True; otherwise, it returns False. Example:
2. `not in` operator: It checks if a value does not exist in a given sequence or collection. If the value is not found, the operator returns True; otherwise, it returns False. Example:
These membership operators can be used with other data structures as well, such as strings, sets, and dictionaries, to check for the presence or absence of specific elements. They are particularly useful when you need to test for the existence of a value within a collection before performing further operations or making decisions in your code. 7. Python Identity OperatorsIn Python, identity operators are used to compare the memory addresses (identities) of two objects. These operators determine whether two objects refer to the same memory location or not. Python provides two identity operators: 1. `is` operator: It checks if two objects refer to the same memory location. If the objects are the same, it returns True; otherwise, it returns False. Example:
2. `is not` operator: It checks if two objects do not refer to the same memory location. If the objects are different, it returns True; otherwise, it returns False. Example:
It's important to note that identity operators compare the objects' memory addresses, not their values. Even if two objects have the same values, they might still have different memory addresses and, therefore, not be considered identical. These operators are useful when you want to determine whether two variables are referring to the exact same object in memory.These are the basic operators in Python. They allow you to perform a wide range of operations on different types of data, such as numbers, strings, and Boolean values. |
What's Your Reaction?
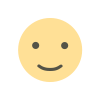
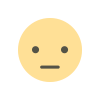
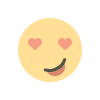
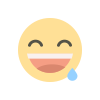
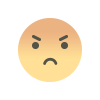
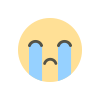
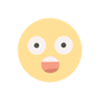