Unraveling the Power of Python Variables: Your Key to Data Mastery-w9school
Discover the significance of Python variables and their role in data manipulation. Dive into Python's variable magic today!
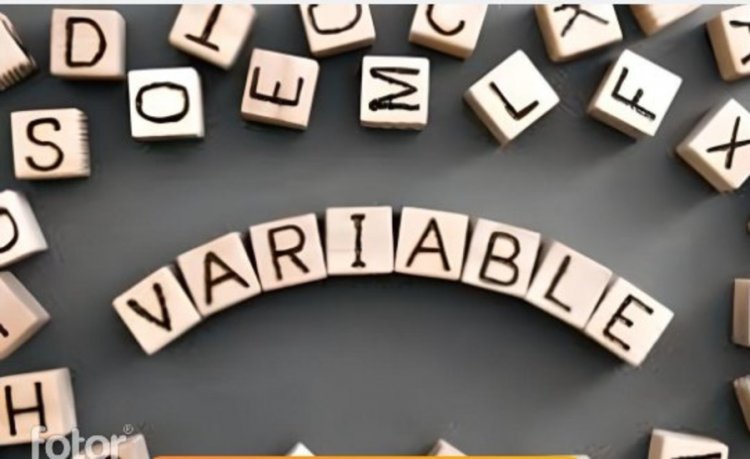
Python Variables |
Variables serve as storage containers for various data values. Creating VariablesIn Python, there is no specific command for declaring variables. However, when you assign a value to a variable for the first time, it is automatically created. Example
There is no requirement to assign a particular type to variables, and they may even alter their type after initialization. Example
|
CastingWhen it comes to programming, the act of defining the data type of a variable is referred to as casting. Example
|
Get the TypeIf you need to know the data type of a variable, the type() method is a great option to consider. Example
|
Single or Double Quotes?You can declare string variables by using either single or double quotation marks. Example
|
Case-SensitiveIt is important to pay attention to the case of variable names. ExampleThis will create two variables:
|
Variable NamesWhen it comes to naming variables in Python, certain rules must be followed.
ExampleLegal variable names:
|
Reminder: The variable names are case-sensitive. |
Multi Words Variable NamesIt can be challenging to comprehend variable names that have more than one word. However, there are several methods that you can use to enhance their readability.
The decision between a snake case or camel case is something that is a matter of personal preferences for projects. The official style guide for Python, PEP 8, recommends using snake_case to define variable and function names, and using CamelCase for names for classes. Consistency in name conventions is crucial to ensure that code is readable and maintainable. |
Many Values to Multiple VariablesIn Python, you can assign values to many variables in a single line: Example
Test YourselfBy now, you should have a good understanding of variables and their usage in Python. Are you feeling confident and ready to apply your knowledge? |
What's Your Reaction?
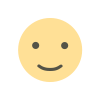
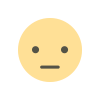
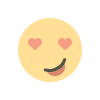
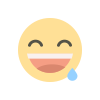
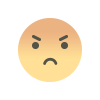
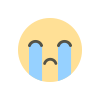
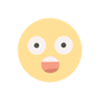