From Strings to Sets: Navigating Python's Data Type Landscape - w9school
Explore the diverse world of Python data types in our comprehensive guide. From strings to sets, navigate the landscape with insights, tips, and practical examples.
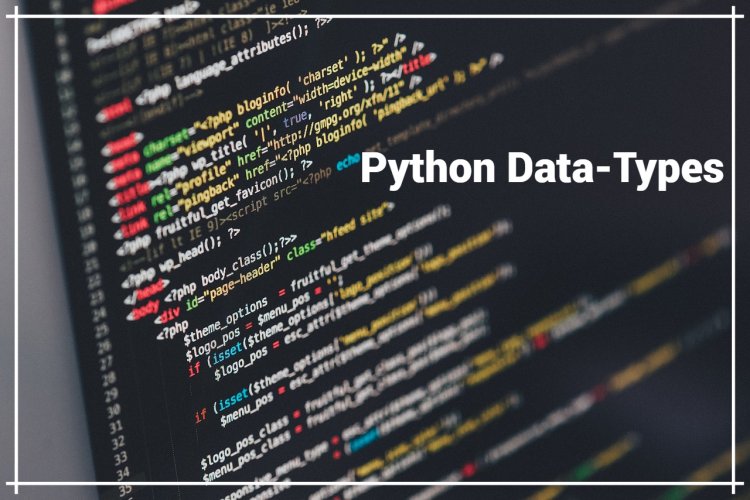
Python Data Types |
In Python, a data type is a classification or categorization of the type of data that a variable can hold. It defines the kind of value that a variable can store and the operations that can be performed on that value. Data types help the Python interpreter understand how to interpret and manipulate the data stored in variables. Python is a dynamically typed language, which means you don't need to explicitly declare the data type of a variable when creating it. Instead, the data type is determined automatically based on the value assigned to the variable. In Python, data types are a way of classifying different types of data that can be used in variables and objects. Python is a dynamically typed language, which means you don't need to explicitly specify the data type when declaring a variable; it is determined automatically based on the assigned value. Here are some commonly used data types in Python:
Python is a dynamically typed language, which means you don't need to declare the data type explicitly when creating variables; it is determined automatically based on the value assigned to the variable. Understanding data types in Python is crucial for effective programming and data manipulation. Each data type has its own properties and methods, which allow you to perform specific operations on the data stored in variables of that type. |
Why we Need Data Types In Python?Data types are essential in programming because they serve several important purposes:
Overall, using data types is an essential aspect of writing robust, efficient, and maintainable Python code. It enables you to work with data more effectively, prevent bugs, and ensure that your programs behave as intended. |
Built-in Data TypesData type is an important notion in programming. Variables can store several types of data, and different types can perform various functions. Python includes the following data types by default in these categories:
|
Getting the Data TypeThe type() function provides the data type of any object: ExamplePrint the variable x data type:
|
Setting the Data TypeWhen you give a value to a variable in Python, the data type is determined:
|
What's Your Reaction?
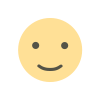
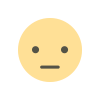
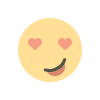
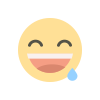
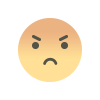
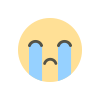
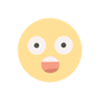