Python Exceptions: Demystifying Error Handling - w9school
Explore Python's exception handling with ease! Unravel the secrets of error management in this comprehensive guide.
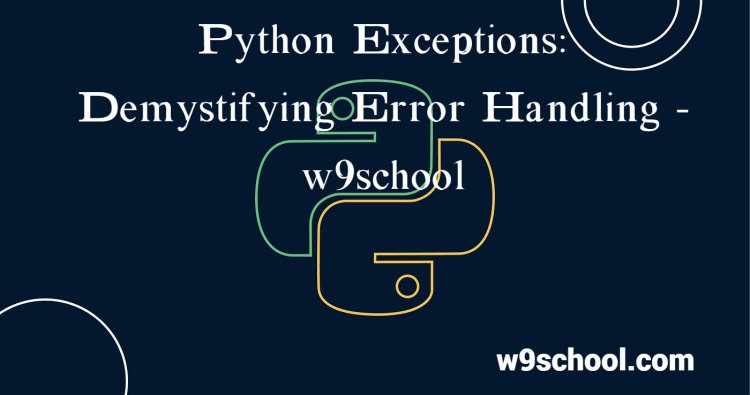
Python Exception Handling |
Python provides us with two basic error management tools—try and except blocks—for handling errors. Use these blocks to isolate problematic code sections, log notifications, or raise exceptions. Create custom exceptions to pinpoint project-specific errors. For instance, when building a grade book app, you can raise GradeValueError instead of ValueError to highlight errors that arise from it.
Try BlockA try block allows you to test a piece of code for errors. When an exception occurs during a try block, Python usually stops and generates an error message; you can add catch blocks that handle these exceptions and finally blocks which continue regardless of their outcome. Code within a try block may generate any number of exceptions; to prepare for anticipated issues, consider including an except block for it in your try block. In this sample code, IndexError, KeyError and FileNotFoundError could all arise during its execution. Your try block can accommodate multiple except blocks; their hierarchy depends on which you define first. For instance, if you want to handle a very specific exception first, place it at the top; otherwise add generic exceptions at the end. Else BlockThe "else" statement (also known as an "elif" statement in Python) allows you to check multiple conditions after an initial "if" statement. Proper indentation of nested if-else blocks is important in maintaining correct structure and execution flow. In the above example, the first if-elif ladder checks if x is greater than 5 and doesn't match. Since its value of 3 isn't, so the code block under the if statement will not execute. Conversely, since its value of 10 does match, then code blocks under either else statements will execute instead. Python will evaluate each if and elif statement until one or more evaluate to True, before continuing with any remaining if-else statements in your program. These can be found within compound statements like if, while, and for loops as well as try block methods. Finally BlockThe finally block allows you to run sections of code that should always run, regardless of unhandled exceptions. This feature can be helpful for performing cleanup tasks such as closing open files and freeing resources, among other purposes. These statements are executed after any try/except statements but before any return, break or continue statements are executed. However, unlike try and except blocks, the finally block does not permit control transfer statements to exit it. If an exception occurs within it that cannot be caught by any catch block it will be raised again as soon as execution has completed; otherwise it continues normally. For instance in this code if an exception occurs during addition of two numbers and does not get caught by try/except blocks then Python would generate an 'DivideByZeroException' error message but finally block ensures division process completes successfully by raising error immediately after finally block has finished execution executing and otherwise executes as normal executing as normal ensuring division process completion successfully. Raise ExceptionPython provides built-in exceptions that cover a broad array of errors and exceptional situations; however, custom exceptions can often be more useful to signal specific conditions within code. You can do this using the raise statement. The raise statement includes an optional from clause, which allows you to chain exceptions together. Its argument must evaluate to an exception class or instance, while also setting its __cause__, __suppress_context__ and __notes__ attributes. When an exception is raised, its execution halts until higher-level exception handlers can be located - this process is known as exception propagation and it is one of the primary purposes for using try... except blocks in your code. A stack backtrace is printed if an exception goes unhandled, showing all active function calls within its current branch of execution - useful when debugging your program and pinpointing any problems; consequently you should always take precautionary measures when creating and handling exceptions in your program. |
Python Exception Handling |
The The The The |
Error handling |
When an error occurs, known as an exception in Python, the program will often halt and produce an error message. The Example The
Given that the Example This statement will result in an error due to the absence of a definition for variable
|
Numerous exceptions |
To handle specific types of errors, you have the ability to create multiple exception blocks. This allows you to execute a particular block of code for a specific kind of mistake. Example Display a single message if the
|
Else |
The "else" keyword can be utilized to specify a code block that will be performed only if no errors were raised: Example In this case, the
|
Finally |
The Example This can be advantageous for terminating objects and managing resources:
Example Attempt to access and modify a file that lacks write permissions:
The application can proceed without keeping the file object open. |
Throw an exception |
As a Python developer, you have the option to raise an exception when a condition happens. To initiate an exception, use the Example Throw an exception and terminate the program if the value of
The Example Throw a
|
For more information,Please visit Home
What's Your Reaction?
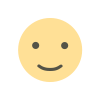
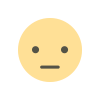
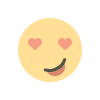
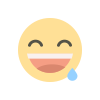
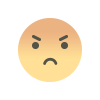
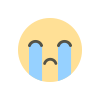
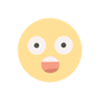