Mastering Python Lists: Your Roadmap to Data Organization - w9school
"Unlock the potential of Python lists for efficient data organization in our comprehensive guide to mastering lists."
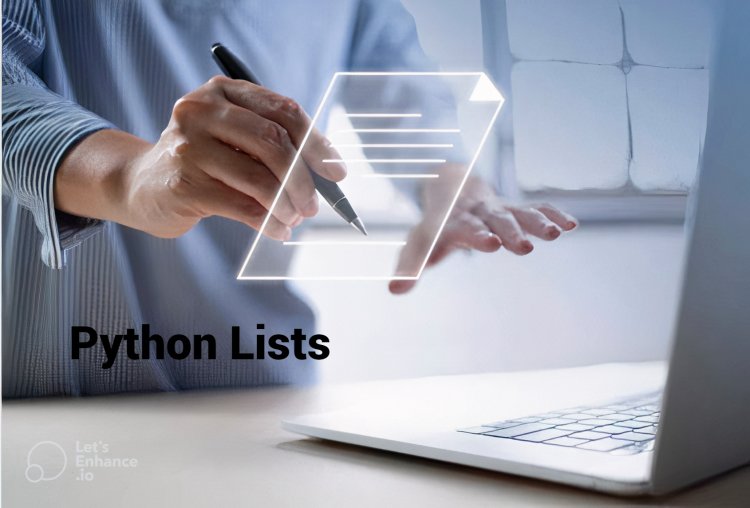
Python Lists |
In Python, a list is a built-in data structure used to store a collection of items. It is a mutable, ordered sequence of elements enclosed in square brackets `[ ]`, where each element is separated by a comma. Lists can contain elements of different data types, including numbers, strings, booleans, or even other lists. Here's an example of creating a list:
Key features and operations associated with Python lists include: 1. Accessing Elements: Elements in a list can be accessed using their index, starting from 0. You can retrieve an element by specifying its index in square brackets `[]`. Example:
2. Modifying Elements: Lists are mutable, meaning you can change or update their elements by assigning new values to specific indices. Example:
3. List Length: You can determine the number of elements in a list using the `len()` function. Example:
4. List Operations: Lists support various operations such as concatenation using the `+` operator and repetition using the `*` operator. Example:
5. List Methods: Python provides several built-in methods for manipulating lists. Some common methods include `append()`, `insert()`, `remove()`, `pop()`, `sort()`, and `reverse()`, among others. Example:
Python lists offer flexibility and versatility for storing and manipulating collections of data. They are widely used due to their dynamic nature and support for a range of operations and methods. |
In Python, Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets: ExampleCreate a List:
List ItemsList items are ordered, changeable, and allow duplicate values. List items are indexed, the first item has index OrderedWhen we say that lists are ordered, it means that the items have a defined order, and that order will not change. If you add new items to a list, the new items will be placed at the end of the list.
ChangeableThe list is changeable, meaning that we can change, add, and remove items in a list after it has been created. Allow DuplicatesSince lists are indexed, lists can have items with the same value: ExampleLists allow duplicate values:
List LengthAs above mentioned, To determine how many items a list has, use the ExamplePrint the number of items in the list:
List Items - Data TypesList items can be of any data type: ExampleString, int and boolean data types:
|
type()From Python's perspective, lists are defined as objects with the data type 'list': <class 'list'>
ExampleWhat is the data type of a list?
|
The list() ConstructorIt is also possible to use the list() constructor when creating a new list. ExampleUsing the
|
Python Collections (Arrays)There are four collection data types in the Python programming language:
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security. |
Python - Access List Items |
Access ItemsList items are indexed and you can access them by referring to the index number: ExamplePrint the second item of the list:
Negative IndexingNegative indexing means start from the end
ExamplePrint the last item of the list:
Range of IndexesYou can specify a range of indexes by specifying where to start and where to end the range. When specifying a range, the return value will be a new list with the specified items. ExampleReturn the third, fourth, and fifth item:
By leaving out the start value, the range will start at the first item:
Range of Negative Indexes
|
Check if Item ExistsTo determine if a specified item is present in a list use the ExampleCheck if "apple" is present in the list:
|
Python - Change List Items |
Change Item ValueTo change the value of a specific item, refer to the index number: ExampleChange the second item:
Change a Range of Item ValuesTo change the value of items within a specific range, define a list with the new values, and refer to the range of index numbers where you want to insert the new values: ExampleChange the values "banana" and "cherry" with the values "blackcurrant" and "watermelon":
If you insert more items than you replace, the new items will be inserted where you specified, and the remaining items will move accordingly: ExampleChange the second value by replacing it with two new values:
If you insert less items than you replace, the new items will be inserted where you specified, and the remaining items will move accordingly: ExampleChange the second and third value by replacing it with one value:
|
Python - Add List Items |
Append ItemsTo add an item to the end of the list, use the append() method: ExampleUsing the
Insert ItemsTo insert a new list item, without replacing any of the existing values, we can use the The ExampleInsert "watermelon" as the third item:
Extend ListTo append elements from another list to the current list, use the ExampleAdd the elements of
The elements will be added to the end of the list. |
Add Any IterableThe ExampleAdd elements of a tuple to a list:
|
Python - Remove List Items |
Remove Specified ItemThe ExampleRemove "banana":
If you do not specify the index, the
|
Python - Loop Lists |
Loop Through a ListYou can loop through the list items by using a ExamplePrint all items in the list, one by one:
|
Python - List Comprehension |
List ComprehensionList comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list. Example: Based on a list of fruits, you want a new list, containing only the fruits with the letter "a" in the name. Without list comprehension you will have to write a Example
|
Python - Sort Lists |
Sort List AlphanumericallyList objects have a ExampleSort the list alphabetically:
ExampleSort the list numerically:
Sort DescendingTo sort descending, use the keyword argument ExampleSort the list descending:
Example
Sort the list descending:
|
Python - Copy Lists |
Copy a ListYou cannot copy a list simply by typing There are ways to make a copy, one way is to use the built-in List method ExampleMake a copy of a list with the
Another way to make a copy is to use the built-in method ExampleMake a copy of a list with the
|
Python - Join Lists |
Join Two ListsThere are several ways to join, or concatenate, two or more lists in Python. One of the easiest ways are by using the ExampleJoin two list:
Another way to join two lists is by appending all the items from list2 into list1, one by one: ExampleAppend list2 into list1:
Or you can use the ExampleUse the
|
Python - List Methods |
List MethodsPython has a set of built-in methods that you can use on lists.
|
Test Yourself With ExercisesNow you have learned a lot about lists, and how to use them in Python. Are you ready for a test? If Yes, then Click Here |
What's Your Reaction?
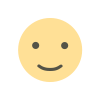
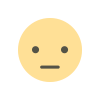
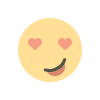
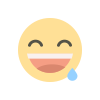
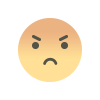
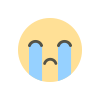
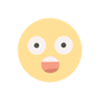