Python Scope Demystified: Understanding Variable Reach
Explore Python scope details master variable visibility and discover the nuances of code functionality. Explore our extensive guide for more in-depth information.
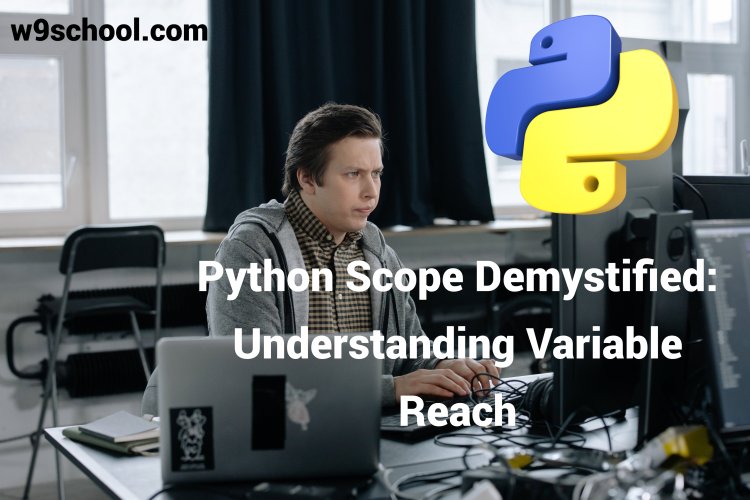
Python Scope |
A variable can only be accessed within the area in which that it is derived from. This is referred to as the scope. |
Levels of Python Scope |
Python provides four levels of access: Local-Enclosing-Global-Builtin. Access depends upon its location within the code. Python creates an internal dictionary when you declare a name, mapping its scope. Each time you reference or access one of those names, Python checks its internal dictionary for its value and looks up what the value might be. Enclosing scopePython provides four variable scopes - Local, Enclosing, Global and Built-in - where variables are created and accessed, providing closures (functions that remember their parent function's variables) or creating closures. Each has their own advantages and disadvantages that need to be taken into consideration when making decisions about variable usage. When names are referenced within a program, they must first be made accessible by its code. Otherwise, an error message will result due to different parts of a program potentially altering them unexpectedly; to prevent this problem from arising further, scoping provides limited access to certain names. Python offers a unique global scope called module scope, meaning each name in a script is only available within its module of definition. This helps keep code organized and prevent conflicts over names between modules. Understanding Python's various scope levels will enable more effective writing. Global scopeA variable's scope defines its access within Python code. When its name is invoked, Python searches in different levels of scope called namespaced regions for it in order to retrieve or assign it; these regions include local, enclosing, global and built-in scopes. Alternatively, if an entry cannot be found it will be searched at each level of scope. For example if using a local variable named x within a function the search will continue in both local and global scopes until either can find it and return an error message if neither does. The nonlocal statement tells Python that names can be modified outside their scope even without calling their respective function, which can help avoid subtle errors when writing programs. As shown below, an example of this concept includes trying to access local variables from outside their scopes via global scopes; when this fails, an error stating they cannot modify local variables due to SyntaxError messages being displayed instead. Built-in scopePython's built-in scope is an arbitrary namespace reserved for preinstalled functions and modules, rather than your code itself. Use of it should be avoided at all costs, as its use can lead to subtle errors that otherwise would remain undetected. Python will generate an error if you try to access variables outside their local scope, because these variables are only visible within their enclosing code block and thus cannot be seen by other parts of your program. To avoid these errors, always utilize the local Python scope. Doing so allows for cleaner and easier-to-read code that's easier to debug and read, plus the LEGB rule helps avoid namespace conflicts when Python references a variable that way! It helps prevent name collisions while writing clean and maintainable code that's easily maintainable! Local scopeThe local scope is a special Python scope that stores names defined within functions. This scope is created at each function call rather than at function definition time and can hold many different names at the same time; additionally, it may even contain subroutines or even nested functions. If you attempt to access an undefined name within the local scope, an error will occur as it can only be accessed within its assigned or defined context. At any one time, there will be at most four Python scopes active: local, enclosing, global and built-in scopes. Python uses the LEGB rule for name resolution in nested functions - searching first for variables in their local scope before expanding this search to global and built-in ones. |
Local Scope |
A variable that is created within an application is within the local area of the function and is only accessible in that specific function. Example A variable that is created in the function is accessible within the function:
Function Inside FunctionIn the previous example The variables x in the example above are not available outside of the function. Example The local variable may be accessible from a function within the function:
|
Global Scope |
A variable that is created within the main body of Python script is an global variable that is part of the scope of global. Global variables can be accessed at any time including local and global. Example Variables that is created without an operation can be global and utilized by anyone:
Naming VariablesIf you use the same name for a variable inside as well as outside of the function Python will consider them to be two distinct variables: one within its global scope (outside within the scope of function) as well as one that is available within the scope local (inside of the program): Example The function prints locally the
|
Global Keyword |
If you want to set up an international variable however, and you're stuck within an area of localization, then you can make use of to use the A Example If you choose to use global keywords, then you are using the
Additionally, you can use also the Example To alter values of global variables within an application, use the variable's name using the
|
For more informative blogs, Please visit Home
What's Your Reaction?
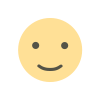
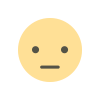
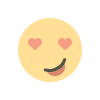
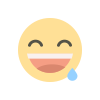
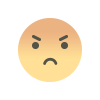
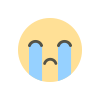
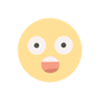