PHP Variables: Unleash Dynamic Programming! - w9school
Explore the power and versatility of Python variables in our latest blog post. Learn how they enable dynamic programming and boost your coding skills.
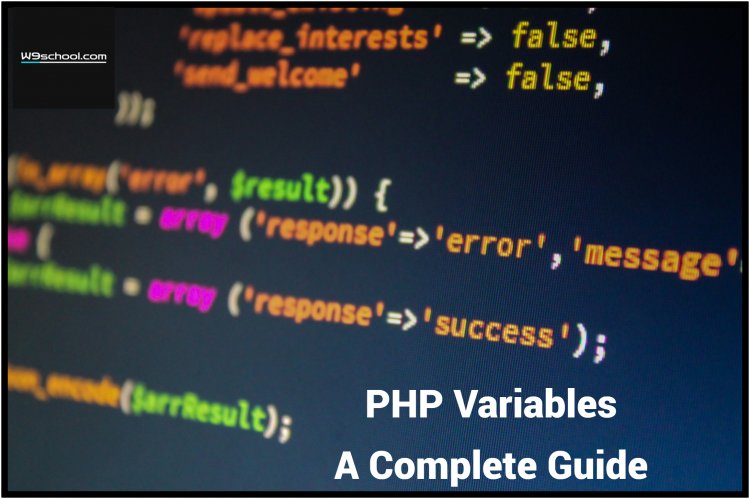
PHP Variables |
PHP variables serve as containers that store information, providing labeled boxes where various objects can be kept. Here are a few key facts about PHP variables:
Strings: Words or sentences enclosed within quotation marks. An example would be: "$name = John;" Numbers: These can be used for counting. For instance, "$age = 10;" means that one's age is 10.
|
Creating (Declaring) PHP VariablesIn PHP variables, variables begin at an Example
After executing these statements following the execution of the statements above, after the execution of these statements, $txt's variable will be holding"Hello world!" The Variable $x is holding the value 5 and that variable will contain the number 10.5.
Consider variables as containers to store information.
PHP Variables NamesA variable may be given a simple name (like the letters x or y) or an evocative name (age carname, total_volume,). The PHP variable rules:
Be aware that PHP variables are case-sensitive! |
Output VariablesIt is the PHP This example will demonstrate what you can do to produce text as an array of variables: Example
In the following instance, you will give identical output to the previous example: Example
This example will show the total of two variables: Example
|
Notification: You will learn more about the echo statement and the way to display data on display in the following chapter. |
PHP is a Loosely Typed LanguageIn the previous example you will notice that we didn't have to inform PHP what data type the variable belongs to. PHP automatically associates a particular data type to the variable based on the value. Because the data types do not have to be defined in the strict sense, it is possible to apply things like adding an integer with a string without creating an error. In PHP 7, declarations of type were added. This allows you to specify the type of data that is required when defining a function and if you enable the requirement to be strict that it throws an "Fatal Error" on a type match. |
PHP Variables ScopeIn PHP variable declaration, variables can be declared in any script. The definition of a variable refers to the area of script that allows the variable to be used or referenced. PHP is a variable PHP that has three areas:
Global and Local ScopeA variable defined in the absence of of a function is within the GLOBAL scope and is able to be used outside of the function ExampleGlobal scope of the variable:
A variable defined in an application has an LOCAL SCOPE. It is only accessible within the function in question: ExampleLocal scope variable:
It is possible to have local variables that have the same name within various functions because local variables can only be recognized by the function for the function in which they are defined. PHP is the global keyword
Global keyword is used to access global variables. In order to do this, add to do this, use the Example
PHP additionally stores the global variables of all PHP users in an array referred to as This example can be modified to read: Example
|
PHP The static KeywordIn general, once a function has been completed or executed, all its variables are removed. Sometimes, we would like to prevent a local variable from be removed. This is to perform a different task. In order to do this, make use of your Example
When the function is invoked this variable will be able to hold the data it had at the time that it was called. |
Note: The variable is not yet local to the program. |
PHP Excersise |
Test Yourself With Exercises
Practice your PHP skills Here
What's Your Reaction?
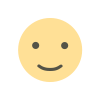
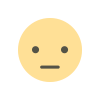
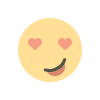
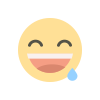
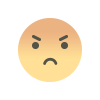
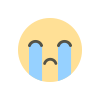
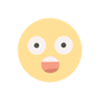