Learning Python Else if: Complete Guide for Beginners - w9school
In Python, the if-else statement is used for conditional execution of code based on a certain condition. It allows you to specify different blocks of code to be executed depending on whether a condition is true or false.
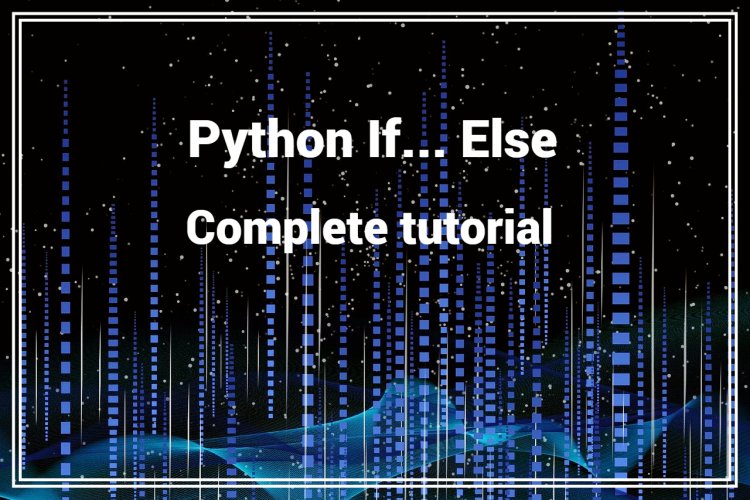
Python If ... Else |
In Python,
|
Usage of If...else in Python |
Here are some common use cases for 1- Simple Conditional Execution:
2- Multiple Conditions:
3-Nested
4- Input Validation:
5- Boolean Conditions:
6- Handling Lists or Iterables:
These are just a few examples of how you can use |
Python Conditions and If statements |
Python supports the usual logical conditions from mathematics:
These conditions can be used in several ways, most commonly in "if statements" and loops. An "if statement" is written by using the if keyword. |
Exampleif statement:
Example Explained: |
Indentation |
Python relies on indentation (whitespace at the beginning of a line) to define scope in the code. Other programming languages often use curly-brackets for this purpose. ExampleIf statement, without indentation (will raise an error):
|
Elif |
The elif keyword is Python's way of saying "if the previous conditions were not true, then try this condition". Example
In this example a is equal to b, so the first condition is not true, but the elif condition is true, so we print to screen that "a and b are equal". |
Else |
The else keyword catches anything which isn't caught by the preceding conditions. Example
|
Short Hand If |
If you have only one statement to execute, you can put it on the same line as the if statement. |
Short Hand If ... Else |
If you have only one statement to execute, one for if, and one for else, you can put it all on the same line: ExampleOne line if else statement:
|
This technique is known as Ternary Operators, or Conditional Expressions. |
You can also have multiple else statements on the same line: ExampleOne line if else statement, with 3 conditions:
|
And |
The and keyword is a logical operator, and is used to combine conditional statements: ExampleTest if
|
Or |
The ExampleTest if
|
Not |
The ExampleTest if
|
Nested If |
You can have Example
|
The pass Statement |
|
Test Yourself With Exercises:
If you are ready to test your skills then Click Here
What's Your Reaction?
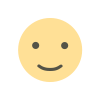
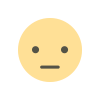
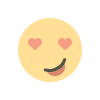
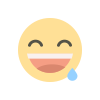
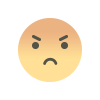
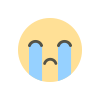
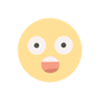