Strings in Python: Your Pathway to Text Manipulation Mastery - w9school
Python strings are sequences of characters, versatile and essential data types for text processing. They support manipulation and formatting with ease.
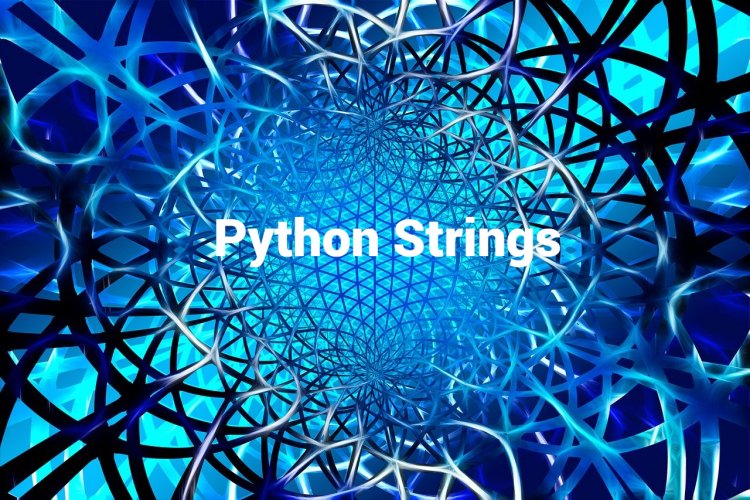
Python Strings |
StringsPython uses either single quotation marks or double quotation marks for defining strings. 'hello' is the same as "hello". You can display a string literal with the |
Assign String to a VariableThe variable name, an equal sign, and the string are used to assign a string to the variable: |
Multiline StringsA multiline string can be assigned to a variable by enclosing it in three quotes: ExampleYou may use three double quotation marks:
|
Note: Please take note that the line breaks in the result are placed exactly where they are in the code. |
Strings are ArraysPython's strings, like those of many other widely used programming languages, are collections of bytes that represent unicode characters. Python does not, however, support character data types; instead, a single character is represented as a string with length 1. To access the string's constituents, use square brackets. ExampleGet the character in position 1 (remember, the first character is in position 0):
|
Looping Through a StringSince strings are arrays, we can loop through the characters in a string, with a ExampleLoop through the letters of the word "banana":
|
String LengthTo get the length of a string, use the ExampleThe
|
Check StringTo check if a certain phrase or character is present in a string, we can use the keyword ExampleVerify the word "free" appears in the following text:
|
Check if NOTTo check if a certain phrase or character is NOT present in a string, we can use the keyword ExampleVerify that the following text DOES NOT contain the word "expensive":
Use it in an
|
SlicingThe slice syntax allows you to return a range of characters. To return a portion of the string, enter the start index and the end index, separated by a colon. ExampleThe characters from positions 2 to 5 (not included) should be obtained:
|
Note: The first character has index 0. |
Slice From the StartIf the start index is omitted, the range's beginning character is the first one: ExampleGet the characters (not included) from position 1 to position 5:
|
Slice To the EndThe range will extend to the end if the end index is omitted: ExampleGet the characters starting at position 2 and continuing to the very end:
|
Negative IndexingStart the slice from the end of the string using negative indexes:ExampleObtain the characters: "World!" (position -5) from: "o" To, but not including: "d" in "World!" (position -2):
|
Python - Modify StringsThere are several built-in methods in Python that you may apply to strings. |
Upper CaseExampleThe
|
Lower CaseExampleThe
|
Remove WhitespaceWhitespace is the space before and/or after the actual text, and very often you want to remove this space. ExampleThe
|
Replace StringExampleThe
|
Split StringThe ExampleThe
|
String ConcatenationUse the + operator to concatenate, or merge, two strings. ExampleMerge variable
|
|
String FormatWe learned that combining strings and numbers in this way is not possible in the Python Variables chapter. Example
But by utilizing the format() technique, we can combine texts and numbers! The given arguments are formatted using the format() method, which also inserts them into the string in the appropriate placeholders: Example Use the
The format() method accepts an infinite number of arguments, which are added to the appropriate placeholders as follows: Example
You can use index numbers Example
|
Escape CharacterUse an escape character to inserted prohibited characters into a string. Backslashes and the character you want to insert are considered escape characters. A double quotation within a string that is encircled by double quotes is an illustration of an unlawful character: ExampleIf you use double quotes within a string that is enclosed in double quotes, you will receive the following error:
The escape character "\" should be used to resolve this issue: ExampleWhen using double quotes, you are permitted to do so when using the escape character:
|
Escape CharactersPython also uses the following escape symbols:
|
String MethodsYou can use a variety of built-in methods on strings in Python.
|
Test Yourself With ExercisesNow you have learned a lot about Strings, and how to use them in Python. Are you ready for a test? If yes Then Click Here for your Python String Test. |
What's Your Reaction?
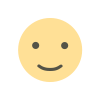
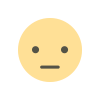
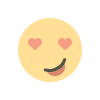
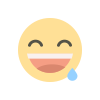
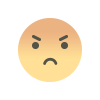
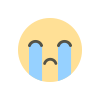
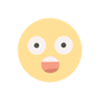