Mastering C++ Conditions: Logic and Decision-Making in Programming - w9school
Explore C++ conditions for effective decision-making. Learn to use if, else, and switch statements. Elevate your programming with robust control flow structures.
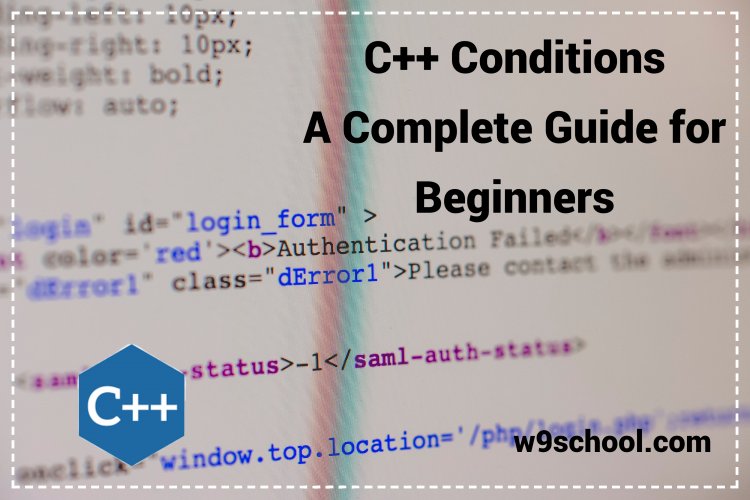
C++ If ... Else |
Conditional Statements in C++C++ is an all-purpose programming language designed for use across many environments, using text editors for writing code and compilers for compiling it into executable programs that your computer understands. There are several compiler options available such as Microsoft Visual Studio; there are also free open source options for Linux and Apple operating systems. C++ offers several conditional statements, with the if statement being the most basic one. This statement checks whether a block of statements will be executed or not according to a boolean value; if true, statements within the curly brackets are executed, otherwise false and they're ignored. The if-else statement is another conditional statement which allows multiple conditions to be checked simultaneously, like an if statement. However, unlike an if statement, all conditions within curly braces must be valid at once in order for this one to work effectively. Switch statements allow you to check multiple cases for one variable at once. They work similarly to an if-else ladder, except they evaluate your expression only once and compare it against all of its values; if an expression matches any one of them then that case's statement will be executed, otherwise the switch statement is ignored and your program continues running normally. |
C++ Conditions and If Statements |
You've already heard the fact that C++ supports the common logical requirements that come from math:
You can make use of these conditions to carry out different actions in different scenarios. C++ has the following conditional statements:
The if StatementUtilize to use the Syntax
|
Take note that |
In the following example we will test two numbers to determine if 20 is greater than. If Example
It is also possible to test the following variables: Example
Example explained In the above example, we will use two factors, x and y to determine if there is a greater value than y for x (using the |
C++ Else |
The else StatementMake use of the Syntax
Example
Example explained In the above example in the above example, the it is clear that the time (20) is more than 18, which means it is |
C++ Else If |
The else if StatementMake use of the Syntax
Example
Example explainedIn the above example it is clear that the it is clear that the time (22) is higher than 10, which means it is clear that the second conditions is If, however, it was the day of 14, the program will write "Good day." |
C++ Short Hand If Else |
Short Hand If...Else (Ternary Operator)Additionally, there is a shorthand If else, also called"the the ternary operator due to its three operands. It is a way to replace several lines of code in one line. It is commonly employed to substitute simple statements like: Syntax
In lieu of writing: Example
It is easy to write: Example
|
Conclusion |
C++ conditions are statements used to decide whether a block of code will run in a program. There are various forms of conditional statements, but perhaps the most widely-used decision-making statement is an if-else statement which checks a condition and executes either one of two blocks of code depending on its result. The if-else statement is an indispensable component of any program. As C++'s most basic form of conditional statements, it enables programmers to check a variety of conditions quickly. You can use multiple or single if-else statements simultaneously; alternatively you may use nested versions. An If-Else statement requires a boolean value to evaluate its condition, which could be an expression, variable, or function call. The value must evaluate to either true or false for proper evaluation; otherwise it will be disregarded and the statement ignored. An if-else statement allows one or both blocks of code to execute, with either block being evaluated depending on whether or not its counterpart executes; if both execute simultaneously, only the second will be evaluated; otherwise both blocks will be disregarded. It can also be combined with another if-then-else statement to evaluate multiple conditions simultaneously. Use a while loop to repeat code until its test condition has been fulfilled, as shown here. In this instance, the while loop will repeat until i is equal to 9. This will display Hello there! on screen 10 times before stopping when its condition has been fulfilled and printing it once more. |
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
Practice your learnings agian and again and be a skillful programmer.
Take test about C++ Conditions and know how much you have actually learnt...
Best of Luck
For more information, Please visit this website
What's Your Reaction?
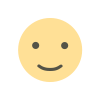
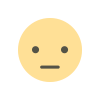
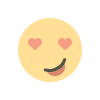
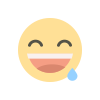
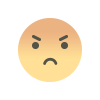
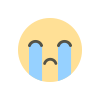
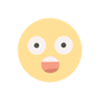