Cracking the Code: Your Ultimate Guide to C++ Variables - w9school
Unleash the power of C++ variables with our ultimate guide. Elevate your coding skills, master variable types, and embark on an epic journey to programming mastery.
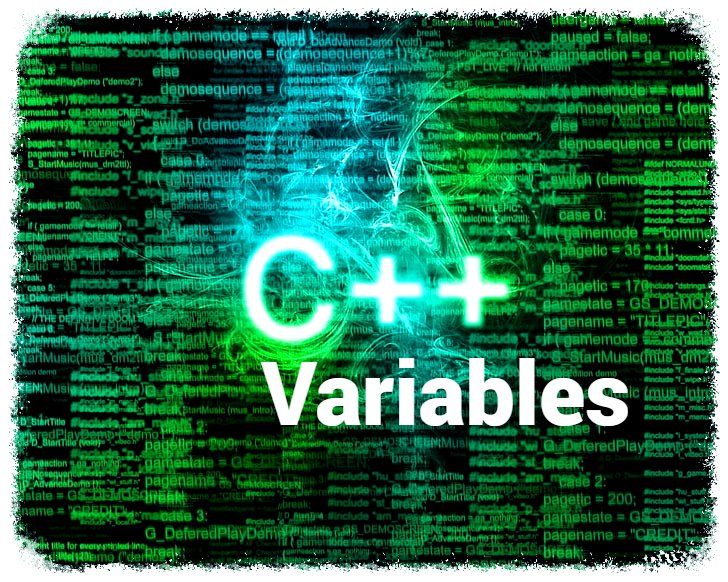
C++ Variables |
Variables are containers that store values for data. Within C++, there are various kinds of variables (defined by different terms) Examples include:
|
Declaring (Creating) Variables |
In order to create variables the type and assign an amount: Syntax
When type is one of the C++ types (such as int) as well as variableName, which is the title given to that variable (such as myName or x). An equal sign equal sign is used to assign values to the variable.
To make a variable which will store a number, consider an example like this: Example Create a variable named myNum of the type
You may also declare a variable, without assigning a value, and assign the value later on: Example
If you give a new value an existing variable replace the value of the previous one: Example
|
Other TypesA display of different types of data: Example
|
Display VariablesThe To mix text and variables Separate them using the Example
|
Add Variables TogetherTo join a variable another variable, utilize an Example
|
C++ Declare Multiple Variables |
Declare Many VariablesTo declare multiple variables of the similar kind make use of a comma separated list: Example
One Value to Multiple VariablesYou can assign the the same value to several variables in one line: Example
|
C++ Identifiers |
Identifiers Each of the C++ variables should need to identified by distinct names.. These names are referred to as identifyrs. Identifiers may be short name (like an x, names like x and) as well as more specific names (age sum, totalVolume,). Notice: It is recommended to utilize descriptive names to produce readable and maintainable code: Example
The most common guidelines for naming variables are:
|
C++ Constants |
Constants If you don't want anyone else (or you) to alter variables' values, utilize your Example
It is best to declare the variable to be constant if the values are likely to change: Example
|
Overview |
Dive into the World of C++ Variables Hey, future coders! Today, we're diving deep into the magic of C++ variables. They're like superheroes in the coding world, and we're about to uncover their secrets. Get ready for an awesome journey into the heart of programming! What's the Deal with Variables? Okay, so variables are like boxes that hold stuff in the computer's brain. Think of them as containers where you keep important info like numbers, words, or even cool data. They're like the Lego bricks of coding – you use them to build amazing things! Let's Start with the Basics In C++, telling the computer you want a variable is super easy. It's like saying, "Hey, computer, I need a box for this special thing." Check it out:
Now you've got a box named 'myNumber' ready to hold some cool numbers. Filling Up Your Box Once you've got your box, it's time to put something inside. This is where the real fun begins. It's like putting your favorite toys in a toy box:
Ta-da! You've just made your variable store a cool number. Take Your Coding to the Next Level Now that you're a variable superhero, go ahead and mix them up. Combine variables to create even cooler stuff:
You're not just coding; you're creating magic! In Conclusion Great job, coding hero! You've just scratched the surface of C++ variables. They're your sidekicks in the coding adventure. Choose wisely, keep it simple, and let your imagination run wild! Happy coding! |
Tips for Super Coding Skills |
Tip #1: Pick the Right Box In C++, you've got different types of boxes for different stuff. Need a box for numbers? Use 'int'. Want one for words? That's 'string'. Choose the right box for your treasures!
Tip #2: Keep Your Boxes Labeled
Imagine a room with boxes, but no labels. Chaos, right? Same goes for coding. Give your boxes good names so you always know what's inside.
|
Exercises |
Test yourself with C++ exercises to check if you have learnt anything or not.. You've only glimpsed into the vast world of C++ variables. Be aware that they're not only boxes. They're your tools for creating incredible things. Therefore, make wise choices and keep it all organized and don't give up! |
What's Your Reaction?
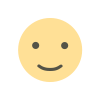
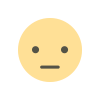
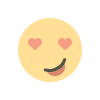
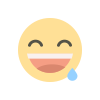
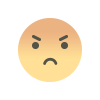
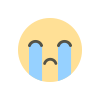
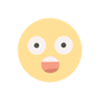