Mastering User Input in C++: A Programmer's Guide - w9school
Dive into C++ user input mastery! Learn clear prompts, error handling, and best practices to enhance your programming skills. Your guide to seamless interaction.
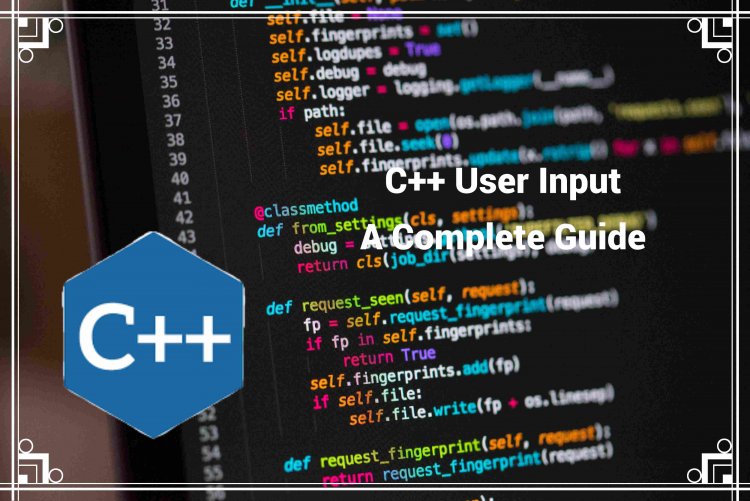
C++ User Input |
We have learned that In the next example, the user is able to enter a number, which can be stored within the variable
C++ can effectively manage user input in various ways:
In the above code, the user's input will be read and stored into the variable
The above code reads a line from the standard input and stores it in the variable
This method can also massively assist with complex user inputs that involve multiple data types.
The above block of code keeps prompting the user until a valid integer is entered. These are the few ways that C++ can be used effectively to manage user input. Always validate user input to avoid any bugs or crashes. |
Good To Know
|
Creating a Simple Calculator |
In this case it is the user's responsibility to enter two numbers. Then, we print the total by taking into account (adding) both numbers Example
Here you are! You've built a simple calculator! |
How can you validate and handle invalid input in C++? |
In C++, you can validate and handle invalid input using various methods. Here are a couple of common strategies:
Remember, always validating and properly handling user input is essential to prevent crashes and errors in your software. |
Conclusion |
In the end, knowing how to efficiently handle input from the user is an essential skill for C++ programming. Utilizing C++ offers various tools for handling input validation, making sure that the program operates in a predictable manner and smoothly in the face of user inputs that are unexpected. State checking of streams, with functions such as It is essential to integrate these methods of validation into your software to prevent crashes and provide a seamless user experience. The examples provided show the loop, which continuously calls the user until they input a valid input. This looping approach allows users to rectify errors in their input, while promoting an intuitive interface for users. In a wider perspective, these ideas go beyond the simple validation of inputs and are crucial to the development of solid software applications. The correct handling of input from users is the most important aspect to developing user-friendly and reliable software. Developers and programmers who are aspiring will greatly benefit from learning these basics, thereby setting the stage for further programming tasks. If you're looking to dive deeper into C++ programming and beyond, browsing other blogs and tutorials could give valuable insight and better understanding of different programming concepts. Keep learning, be curious, and keep programming and check out other blogs to improve your programming abilities. Beginning the journey of learning how to code can open up infinite possibilities. Continuous learning and exploration are the key to mastering every programming language. Enjoy programming! If you found this post informative, you should look into other blogs to increase your knowledge. Have fun coding! |
Test Yourself With Exercises |
If you are ready to test yourself then click Here |
What's Your Reaction?
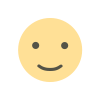
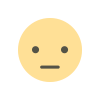
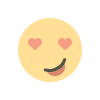
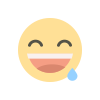
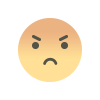
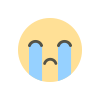
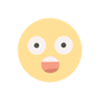