How do I Learn about Python Function? -w9school
In Python, a function is a block of reusable code that performs a specific task. Functions allow you to break down your program into smaller, manageable pieces, making the code more organized, modular, and easier to maintain.
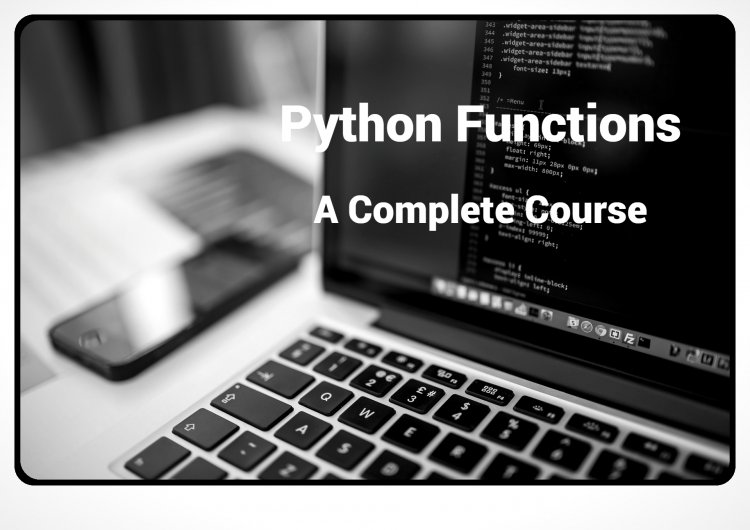
What is a Function in Python? |
A function is a section of code that only executes when called. You can supply parameters—data—to a function. As a result, a function may return data. A Python function is a block of organized, reusable code that performs a specific task. It encapsulates a set of instructions that can take input, process it, and produce output. Functions are a fundamental building block of Python programming, enabling you to create modular and organized code. Functions are an essential concept in programming that allow you to group a set of statements together to perform a specific task. In Python, functions are blocks of reusable code that can take input, perform operations, and produce output. They help in organizing code, promoting code reusability, and making the code more modular and maintainable. Functions can also have default parameter values, variable-length arguments, and keyword arguments, making them quite flexible and versatile. Using functions in Python helps in code organization, reusability, and maintainability. You can define a function once and use it throughout your program, making your code more efficient and easier to manage. |
Basic Structure of a Function |
Here's a basic structure of a Python function:
Let's break down the components:
|
Example of a Simple Function |
Here's a simple example of a function that adds two numbers and returns the result:
In this example, the function ' Python also allows for default parameter values and variable-length arguments through Here's a quick overview: Default Parameter Values: You can assign default values to function parameters. If a value is not provided for that parameter when the function is called, it will use the default value.
Variable-Length Arguments: You can use
Functions are a powerful feature in Python that allow you to write cleaner, more organized code and facilitate code reuse. They play a crucial role in writing efficient and maintainable programs. |
Why Functions are Important in Python |
Functions are crucial in Python, as well as in programming in general, for a variety of reasons. Here are some of the key reasons why functions are important in Python: 1. Modularity and Reusability: Functions allow you to break down a complex problem into smaller, manageable tasks. This promotes code modularity, making it easier to understand and maintain. Once you've written a function, you can reuse it throughout your program or even in other programs, saving time and effort. 2. Code Organization: Functions provide a structured way to organize your code. Instead of having a single large block of code, you can group related operations into separate functions. This makes your codebase more organized and easier to navigate. 3. Readability and Maintainability: Well-named functions with clear purposes improve code readability. When functions have a single responsibility, they are easier to understand and debug. This makes maintaining and updating your code simpler, especially as the codebase grows. 4. Abstraction: Functions allow you to encapsulate complex operations behind a simple interface. This means that you can use a function without knowing all the details of how it works internally. This concept of abstraction helps in reducing complexity and cognitive load. 5. Code DRY (Don't Repeat Yourself): Functions promote the DRY principle by allowing you to write a piece of code once and reuse it multiple times. This reduces redundancy and the chances of introducing errors when making changes. 6. Testing: Functions can be tested individually, which makes it easier to identify and isolate bugs. Writing tests for functions helps catch issues early in the development process and ensures that the functions work as expected. 7. Parameterization: Functions can accept parameters, allowing you to create more generic and flexible code. By changing the input parameters, you can achieve different outcomes without duplicating code. 8. Collaboration: In collaborative programming environments, functions provide a way for team members to work on different parts of a program simultaneously. Each team member can focus on implementing specific functions without interfering with others' work. 9. Standard Library and Third-Party Libraries: Python's standard library and third-party libraries are built using functions. By understanding how functions work, you can leverage these libraries to extend the capabilities of your programs without reinventing the wheel. 10. Debugging: Functions help narrow down the scope of potential issues. If a problem occurs, you can focus on the specific function where the issue originates, making debugging more efficient. In summary, functions are essential in Python because they enhance code organization, maintainability, and reusability. They empower you to write efficient, modular, and readable code, making your programming experience more productive and enjoyable. |
Creating a Python Function |
Function definition in Python is done with the def keyword: Example
|
Calling a Python Function |
Use the function name in parenthesis to call the function: Example
|
Arguments in Python |
Functions accept arguments that can contain data. The function name is followed by parenthesis that list the arguments. Simply separate each argument with a comma to add as many as you like. The function (fname) in the following example only takes one argument. A first name is passed to the function when it is called, and it is utilized there to print the whole name: Example
Parameters or Arguments?Information supplied into a function can be referred to as both a parameter and an argument. From the viewpoint of a function: The variable in the function definition listed between parentheses is referred to as a parameter. When a function is called, a value is supplied as an argument. Number of ArgumentsBy default, the appropriate number of parameters must be sent when calling a function. In other words, if your function requires 2 arguments, you must call it with 2 arguments, not more or fewer. Example
An error will be returned if you try to call the method with just one or three arguments: Example
Arbitrary Arguments, *argsAdd a * before the parameter name in the function specification if you are unsure of the number of arguments that will be given to your function. The function will then receive a tuple of parameters and be able to access the components as necessary: Example The parameter name should be preceded by a * if the number of arguments is unknown:
Keyword ArgumentsThe key = value syntax allows you to send parameters as well. This makes the arguments' chronological order irrelevant. Example
Arbitrary Keyword Arguments, **kwargsBefore the parameter name in the function definition, add two asterisks: ** if you are unsure of the number of keyword arguments that will be provided into your function. This will allow the function to access the items appropriately after receiving a dictionary of arguments: Example If the number of keyword arguments is uncertain, a double ** should come before the parameter name:
Default Parameter ValueThe use of a default parameter value is demonstrated in the example that follows. The function uses the default value if it is called without any arguments: Example
A List Being Passed as an ArgumentA function will handle any data type that is supplied to it as an argument, including strings, numbers, lists, dictionaries, etc. If a List is given as an argument, for example, it will still be a List when it reaches the function: Example
Return ValuesTo enable a function to return a value, use the return statement: Example
The pass Statement
Example
|
Recursion |
Additionally, Python allows function recursion, which lets defined functions call one another. Recursion is a common concept in both arithmetic and programming. It indicates when a function calls itself. The benefit of this is that you can loop through the data to get a conclusion. The developer should exercise extreme caution when using recursion because it is quite simple to write a function that never ends or consumes excessive amounts of memory or processing resources. But when used correctly, recursion may be a tremendously useful and mathematically elegant programming approach. Tri_recursion() is a function that we defined to call itself ("recurse") in this example. The data is the k variable, which decreases by one (-1) with each recursion. When the condition is 0 (that is, when it is 0), the recursion comes to a conclusion. A new developer may need some time to fully comprehend how something works; the best way to do this is to test and modify it. Example Recursion Example
|
Test Yourself With ExerciseNow that you have learnt enough about functions it's time to test yourself. Are you Ready? If Yes then Click Here |
What's Your Reaction?
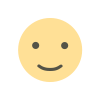
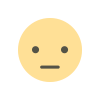
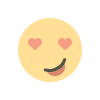
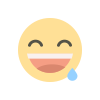
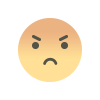
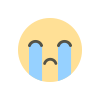
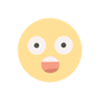