JSON in Python: A Developer's Guide to Effortless Serialization
Dive into the Python JSON realm! Explore seamless serialization, decoding, and data magic. A developer's guide to crafting elegant solutions effortlessly
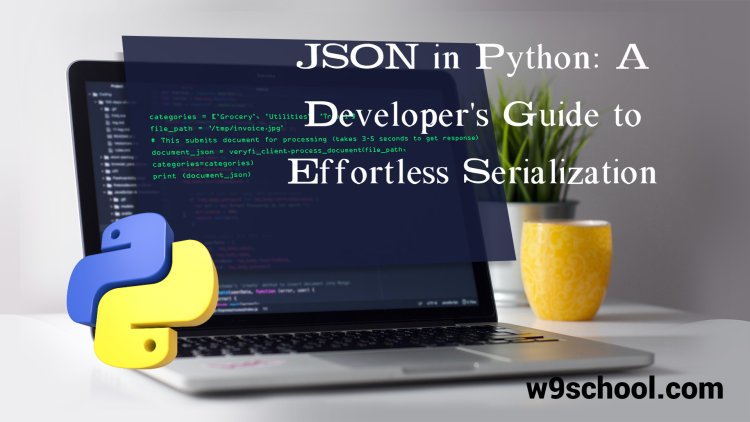
Python JSON |
What is Python JSON?Python JSON provides a powerful way of storing and exchanging data in your program, as well as being an efficient means for building APIs and configuring files. With an intuitive conversion method that easily transforms objects to an easily readable file. JSON is a syntax for storing and exchanging data. JSON is a text format that is written in JavaScript the use of object notation. If check_circular is set to true, lists and dictionaries will be checked for circular references during encoding to avoid infinite recursion. By default, ensure_ascii will also translate all non-ASCII characters to ASCII strings for easier readability.
Python’s JSON modulePython comes equipped with a JSON module that enables you to convert objects from and into JSON strings, providing crucial functionality when working with many modern web APIs. If a string is not JSON, it is converted to an array of integers, floats or bools. Additionally, you can subclass JSONDecoder and override object_hook to add your own custom decoding functions. The JSON module employs indent levels to format array elements and tuples more aesthetically. A level 0 indent will add newlines; none won't introduce any. EncodingPython supports many data formats. One of the most popular is JSON, an easily exchanged data format with its own library for encoding and decoding functions. The default implementation of the encoding method returns either a serializable object for object o, or throws a TypeError. The parameter skipkeys determines whether keys that do not fall under str, int, float or None are encoded; when set to False this setting prevents storing non-ASCII characters in output data. DecodingNo matter whether it is for importing data from an API, transporting it across networks or just organizing code, chances are JSON will play a part. Python makes working with JSON almost ridiculously straightforward. The dumps function decodes strings into Python objects and accepts as arguments a Sort_Keys attribute that specifies which function should be called on each key in JSON data. If the decoder detects keys that do not belong to one of the supported types, such as str, int, long, float, Decimal or bool keys - or do not match an ASCII encoding - as not being strings or integers it will raise a TypeError error and ignore byte sequences not matching an ASCII encoding scheme - an error will be raised as well as being ignored. ObjectsJSON is an easily readable data format designed for humans and machines alike, making it popularly used to exchange information between servers and web applications. Python's JSON module features methods for encoding and decoding custom data types, making it ideal for tasks such as writing configuration files. Skilled at handling JSON data can be an invaluable skill for any developer, particularly when dealing with modern APIs that provide this type of output. VariablesJSON is an ideal format for exchanging data between programs. Utilizing its simple key-value pair structure, JSON is widely employed by web servers and APIs alike. Python's built-in JSON module makes for quick and simple conversion of Python objects to and from JSON strings, including support for URI template escaping mechanisms. Python offers complete support when it comes to JSON encoding, including complex numbers and booleans. Nested dictionary keys enable more complex structures while checking for circular references helps prevent recursion errors during encoding. StringsJSON is an increasingly popular format for transmitting data. It can be utilized by web browsers, APIs and modern databases; understanding its structure and handling are integral parts of programming in Python. Python's JSON module can help transform data from lists or dictionaries into JSON strings, as well as vice versa. This library also has an option to parse nested JSON. However, take caution with backslashes as they are not escaped by default and may cause issues when working with third-party APIs. ArraysArrays are data structures that store values in contiguous regions of memory. They can hold numbers, strings, Boolean values (true/false), objects or even combinations thereof. To access an element in an array, the index serves as the key; this positive integer number enclosed within square brackets should begin counting from zero instead of one; Python starts counting at 0. Therefore it is critical that an accurate index specification be given in order to successfully access all elements within an array. Arrays are extremely beneficial because they allow you to quickly access values in a list without searching through it all for them - this feature is especially useful when working with large amounts of data. XMLXML is a data format that uses tags to organize and describe information. Rather than being a programming language, XML serves as a markup language that describes how data should be stored and transported over the Internet. The JSON module in Python works similarly to marshal and pickle modules by providing functions for reading and writing JSON data, as well as functions to convert between objects and strings and verify documents against schema. It includes validation methods. |
JSON in Python |
Python comes with a built-in program named Example Import the JSON module:
Parse JSON - Convert JSON to PythonIf you've got an JSON string that you want to parse, you can do so using the |
||||||||||||||||||||
The end result will be the result will be a Python dictionary. |
||||||||||||||||||||
Example Convert JSON in to Python:
Convert Python to JSON If you own an Python object that you want to convert, you can turn it to an JSON string making use of the Example Convert Python into JSON:
It is possible to convert Python objects, which fall into the following types, to JSON strings:
Example Convert Python objects into JSON strings and print the value:
If you are converting from Python in to JSON, Python objects are transformed to JSON equivalents. JSON (JavaScript) equivalent
Example Convert an Python object to contain all legal types of data:
|
Format the Result |
The image above displays the JSON string, however, it's not a breeze to read. There are no lines or indentation. It is the Example Make use of to use the
You can also specify the separators. The default value can be (", ", ": "), which is an apostrophe and a space to separate the objects as well as a colon and an asterisk to distinguish the keys from the values: Example Make use of the
|
Order the Result |
This Example Make use of the
|
For more information, Please visit Home
What's Your Reaction?
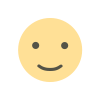
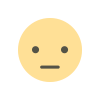
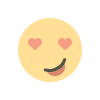
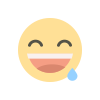
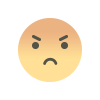
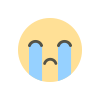
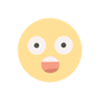