Unlocking the Magic of Python Dictionaries: Your Ultimate Beginner's Guide- w9school
Master Python dictionaries effortlessly! Explore key-value wonders in this ultimate beginner's guide. Start your Python journey today.
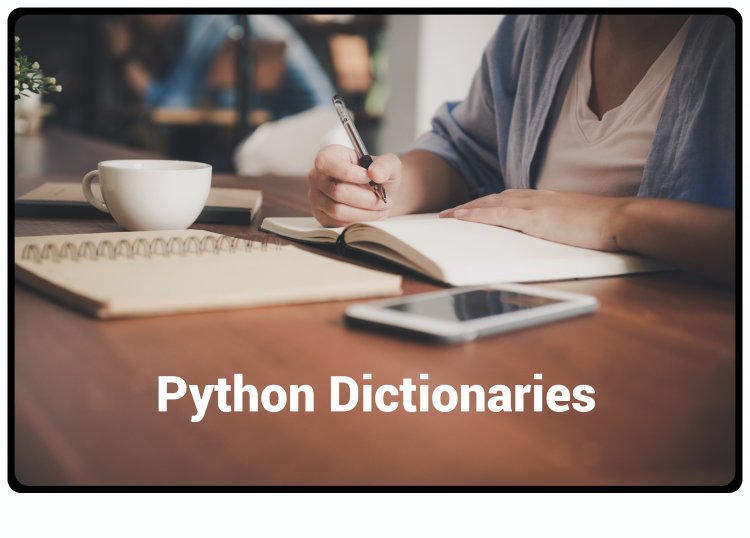
Python Dictionaries |
DictionaryPython dictionaries are data structures that store key-value pairs. They are also known as associative arrays or hash maps in other programming languages. Python dictionaries are mutable, unordered, and can be nested. Python dictionaries are used to store data values in key:value pairs. A Python dictionary is a collection which is ordered*, changeable and do not allow duplicates. As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered. In Python dictionaries, keys must be unique and immutable (strings, numbers, or tuples). Values can be of any data type (strings, numbers, lists, dictionaries, etc.). Python dictionaries are commonly used to store and retrieve data based on specific keys, allowing for efficient lookup operations. In Python, dictionaries are written with curly brackets, and have keys and values: ExampleCreate and print a dictionary:
Python Dictionary ItemsPython dictionary items are ordered, changeable, and does not allow duplicates. Python dictionary items are presented in key:value pairs, and can be referred to by using the key name. ExamplePrint the "brand" value of the dictionary:
Ordered or Unordered?As of Python version 3.7, Python dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered. When we say that python dictionaries are ordered, it means that the items have a defined order, and that order will not change. Unordered means that the items does not have a defined order, you cannot refer to an item by using an index. ChangeablePython dictionaries are changeable, meaning that we can change, add or remove items after the dictionary has been created. Duplicates Not AllowedPython dictionaries cannot have two items with the same key: ExampleDuplicate values will overwrite existing values:
Python Dictionary LengthTo determine how many items a python dictionary has, use the Python dictionary Items - Data TypesThe values in dictionary items can be of any data type: ExampleString, int, boolean, and list data types:
|
type() |
From Python's perspective, dictionaries are defined as objects with the data type 'dict':
ExamplePrint the data type of a dictionary:
The dict() ConstructorIt is also possible to use the dict() constructor to make a dictionary. ExampleUsing the dict() method to make a dictionary:
|
Python Collections (Arrays) |
There are four collection data types in the Python programming language:
|
*Set items are unchangeable, but you can remove and/or add items whenever you like. |
**As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered. |
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security.
Python - Access Dictionary Items |
Accessing ItemsYou can access the items of a dictionary by referring to its key name, inside square brackets: ExampleGet the value of the "model" key:
There is also a method called
get() that will give you the same result:ExampleGet the value of the "model" key:
Get KeysThe ExampleGet a list of the keys:
The list of the keys is a view of the dictionary, meaning that any changes done to the dictionary will be reflected in the keys list. ExampleAdd a new item to the original dictionary, and see that the keys list gets updated as well:
|
Python - Change Dictionary Items |
Python - Add Dictionary Items |
Python - Remove Dictionary Items |
Removing ItemsThere are several methods to remove items from a dictionary: ExampleThe
ExampleThe
ExampleThe
ExampleThe
ExampleThe
|
Python - Loop Dictionaries |
Loop Through a Python DictionaryYou can loop through a dictionary by using a When looping through a python dictionary, the return value are the keys of the python dictionary, but there are methods to return the values as well. ExamplePrint all key names in the dictionary, one by one:
ExamplePrint all values in the dictionary, one by one:
ExampleYou can also use the
ExampleYou can use the
ExampleLoop through both keys and values, by using the
|
Python - Copy Dictionaries |
Copy a Python DictionaryYou cannot copy a dictionary simply by typing There are ways to make a copy, one way is to use the built-in Dictionary method ExampleMake a copy of a dictionary with the
Another way to make a copy is to use the built-in function ExampleMake a copy of a dictionary with the
|
Python - Nested Dictionaries |
Nested Python DictionariesA dictionary can contain dictionaries, this is called nested dictionaries. ExampleCreate a dictionary that contain three dictionaries:
Or, if you want to add three dictionaries into a new dictionary: ExampleCreate three python dictionaries, then create one python dictionary that will contain the other three python dictionaries:
Access Items in Nested Python DictionariesTo access items from a nested dictionary, you use the name of the dictionaries, starting with the outer dictionary: |
Python Dictionary Methods |
Python Dictionary MethodsPython has a set of built-in methods that you can use on dictionaries.
|
Benefits of using Python-Dictionaries |
Python dictionaries offer several benefits, making them a versatile and powerful data structure. Here are some of the key benefits of using Python dictionaries: 1. Efficient Data Retrieval: Dictionaries provide a fast and efficient way to retrieve values based on their keys. Instead of searching through the entire data structure, dictionaries use a hash function to directly locate the value associated with a given key. This makes dictionary lookups very efficient, even for large datasets. 2. Flexible Key-Value Mapping: Dictionaries allow you to map keys to corresponding values, enabling you to organize and access data in a meaningful way. This flexibility is particularly useful when dealing with complex data structures or when you need to associate specific information with unique identifiers. 3. Dynamic and Mutable: Dictionaries are mutable, meaning you can modify, add, or remove key-value pairs after the dictionary is created. This dynamic nature allows you to update your data as needed, making dictionaries suitable for scenarios where data changes frequently. 4. Versatile Data Types: Python dictionaries can store values of any data type, including numbers, strings, lists, tuples, and even other dictionaries. This versatility allows you to represent complex relationships and structures within a single data structure. 5. No Fixed Order: Dictionaries in Python are unordered, which means the order of elements is not guaranteed. This characteristic is beneficial when you don't need to rely on the sequence of items and prefer faster lookup times over maintaining a specific order. 6. Fast Membership Testing: Checking for the existence of a key in a dictionary is very efficient. Python uses hash tables internally for dictionaries, making membership testing (e.g., using the `in` operator) faster compared to other data structures, especially as the size of the dictionary grows. 7. Efficient Key-Value Manipulation: Python dictionaries provide built-in methods and operations to manipulate key-value pairs. You can easily add new key-value pairs, update existing values, remove items, iterate over keys or values, and perform various operations to manage and transform dictionary data efficiently.
Overall, Python dictionaries offer a powerful combination of efficiency, flexibility, and versatility, making them a go-to choice for organizing and accessing data in Python programs. |
Python Dictionary Exercises |
Test Yourself With Exercises
Now you have learned a lot about python dictionaries, and how to use them in Python.
Are you ready for a test?
If Yes, then Click Here
What's Your Reaction?
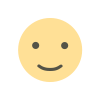
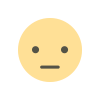
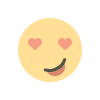
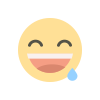
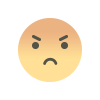
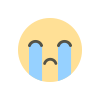
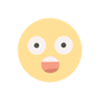