Demystifying C++ Booleans: Data Types and Logic Operators Guide - w9school
Unlock the potential of C++ Booleans! Dive into data logic, grasp Boolean types, and elevate your coding with essential logical operators. Explore true and false in C++ for robust, efficient programming.
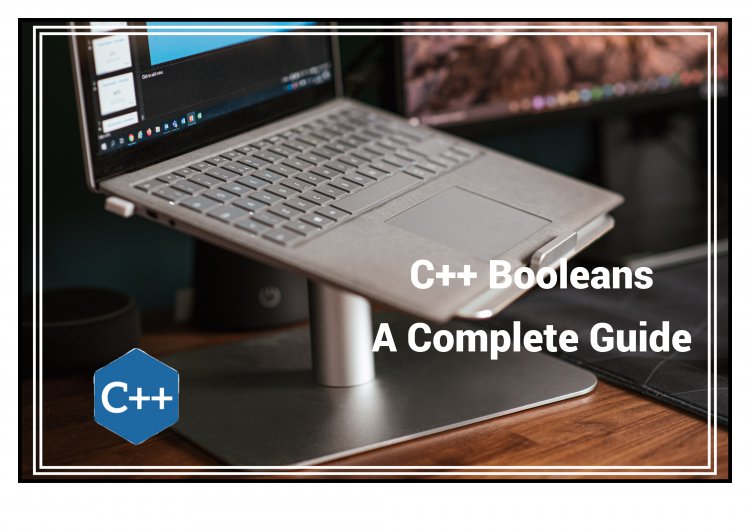
C++ Booleans |
Booleans form the cornerstone of many programming language control structures, from if/else to loops. Utilizing appropriate types for boolean inputs helps maintain clean code that clearly communicates your intentions to callers. Boolean values only offer two states: true or false. Although Booleans seem limited in scope, there are several predefined values which can be assigned to a variable of this data type (referred to as an enumerator or element). Examples may include genre such as rock and jazz music as well as specific items on shopping lists such as bananas or peanut butter. C programming language defines Boolean values with the bool type. You can create variables directly using std::cin functions, but for greater expression use bool as the return type for one of your functions - it will allow you to communicate more precisely your intentions than an int. There are three boolean operators you can use to combine or evaluate boolean expressions: the AND (and), OR (or), and NOT (not). By performing explicit or implicit type conversion, it's also possible to convert non-bool values to bools. Zero is considered false while any non-zero integer or floating point number can be converted using constructs like bool(value) or value!= 0. However, precision constraints do apply so be mindful when making conversions such as this as precision restrictions apply. Furthermore, bools provide memory efficiency as they store only 1 of 2 different values without taking up space with extraneous data. Often, when programming, you'll require a data type that may be limited to two options, for example:
To do this, C++ has an |
Boolean Values |
A boolean value is identified by the Example
In the above example you can see that the It is however more typical to return a boolean number through comparison of the values with variables (See Below). |
C++ Boolean Expressions |
Boolean Expression A Boolean function yields a boolean value that is one of This helps to develop the ability to think and come up with answers. You can employ an comparison Operator for example, like greater than ( >) operator greater than ( Example
Perhaps even more simple: Example
In the following examples we will use in the examples below, we use equal ( Example
Example
|
Real-life Example |
Let's imagine a "real-life example" where we must determine the age of a person to be able to vote. In the following example, we will use an Example
Cool, right? Another option (since we're having a blast right now) is to wrap the code above into the form of an Example Output "Old enough to vote!" If
|
Booleans are the foundation for every C++ comparison and condition. The details about the conditions ( |
How to Avoid Mistakes When Using Booleans in C++ |
|
C++ Exercises |
Test Yourself with C++ exercises you have learnt much about C++ Booleans Now its time to test and check if you can pass this test. To start the test Click Here |
For More Information about Programming languages Visit our website
What's Your Reaction?
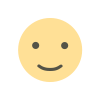
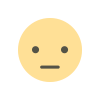
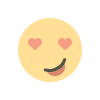
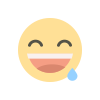
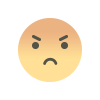
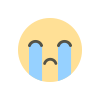
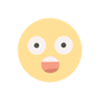