Python Booleans Unveiled: Separating Fact from Fictions - w9school
In Python, booleans are a data type that represents two possible values: True and False. Booleans are used to express logical conditions and are essential for control flow in programming.
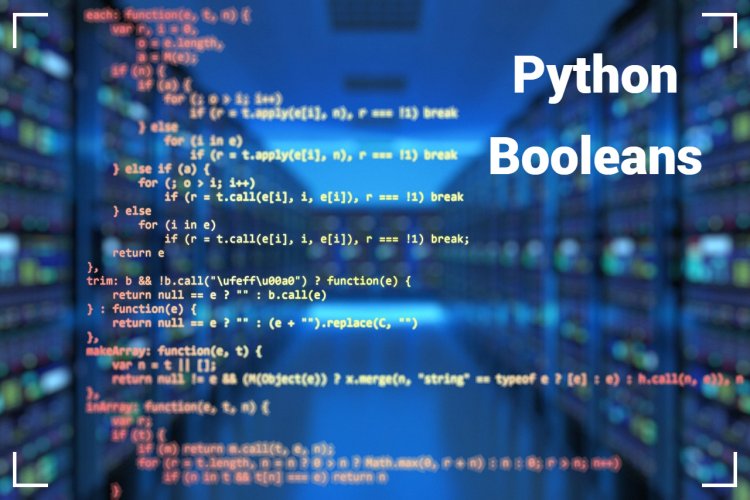
Python Booleans |
In Python, a boolean is a data type that can have one of two values: ' Here's some information about Python booleans: Boolean Values:
Boolean Operators: Python provides several boolean operators that you can use to combine or manipulate boolean values:
Comparison Operators: These operators are used to compare values and return boolean results:
Truthy and Falsy Values: In Python, values that are considered "truthy" evaluate to Conditional Statements: Booleans are commonly used in conditional statements to control the flow of a program. The
Boolean Functions: Python provides built-in functions that work with boolean values:
Ternary Conditional Operator: Python has a concise way to write simple conditional expressions using the ternary operator:
These are some of the key aspects of booleans in Python. They are fundamental for controlling program logic and making decisions based on conditions. |
Why Booleans are Important |
Boolean values in Python (True and False) are fundamental data types that play a crucial role in programming and logic. They serve several important purposes:
In essence, Boolean values are a foundational building block of programming that enable decision-making and conditional execution, allowing you to write code that responds to different situations dynamically. They are used extensively throughout software development for control flow, data filtering, and logical reasoning. |
Boolean ValuesYou frequently need to know whether an expression in programming is True or False. In Python, you may evaluate any expression to see whether it is True or False. When two values are being compared, Python evaluates the expression and returns a Boolean result: Example
Python returns True or False when a condition in an if statement is executed: ExampleDepending on whether the condition is True or False, print a message:
Now Try it Yourself.>> |
Evaluate Values and VariablesAny value can be evaluated using the bool() function, which returns True or False. |
Most Values are TrueAny content in a value is evaluated to True almost instantly if it exists.
ExampleThe following will return True:
|
Some Values are FalseThere aren't many values that evaluate to False, aside from empty values like (), [],, "", 0 and None. The value False, of course, evaluates to False. Example The subsequent will result in False:
If you have an object derived from a class having a '__len__' function that returns 0 or False, one extra value, or object in this example, evaluates to False: Example
|
Functions can Return a BooleanFunctions that return a Boolean Value can be written: Example Print out a function's result:
|
Overview of Python Booleans |
Certainly! Here's an overview of Boolean values and their usage in Python: Boolean Values: In Python, Boolean values are either Boolean Operators: AND: The
OR: The '
NOT: The
Comparison Operators:
Conditional Statements: Conditional statements allow you to execute different code blocks based on conditions.
Boolean Functions: Functions often return Boolean values, such as the
Boolean in Data Structures: Boolean values are often used for filtering elements in lists, comprehensions, and iterating through data.
Boolean values are a fundamental part of programming in Python, used for decision-making, data filtering, and logical operations. They allow you to write code that responds to different conditions and situations in your program. |
Test Yourself With Exercises |
What's Your Reaction?
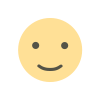
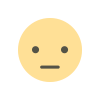
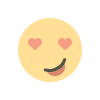
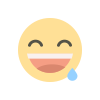
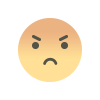
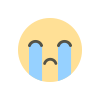
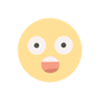