Mastering Python Syntax: Your Essential Guide to Pythonic Perfection-w9school
Unlock the secrets of Python syntax in our comprehensive guide. From basics to mastery, discover the path to Pythonic perfection. Start coding today!
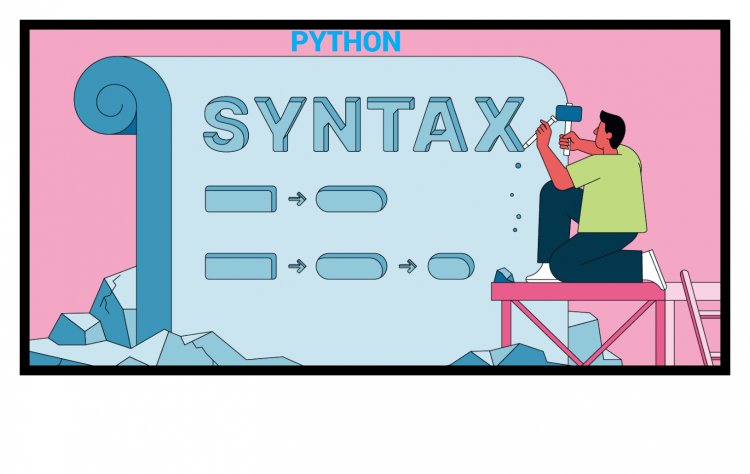
What is Syntax?The syntax of a programming language comprises regulations and principles that govern the arrangement of words, phrases, and punctuation. |
Python SyntaxIt is possible to run Python syntax straight from the command line.
You can generate a Python file on the server with the ".py" file extension, and then execute it through the command line.
|
Python IdentationSpaces at the start of a code line are known as indentations. Unlike other programming languages that use indentation for readability, Python considers it an essential aspect. In Python, indentation is used to indicate a code section
Skipping indentation in Python will result in an error being generated. Example Syntax Error:
When it comes to programming, the decision on how many spaces to use is entirely up to you. However, it's worth noting that the most prevalent amount is four, and it's imperative to use at least one space.
Example
Ensuring that the spacing is consistent within each code block in Python is crucial. Any deviation from this standard may lead to the occurrence of errors.
|
Python VariablesTo create a variable in Python, all you have to do is assign a value to it. It is worth noting that when working with Python programming language, there is no designated command for declaring variables. You will learn more about variables in the Python Variables chapter.
Assigning a value is all it takes to create a variable in Python. |
Comments
Example Comments in Python:
Example
When you add a comment to the end of a line in Python, any text that follows the "#" symbol will not be considered by the program. This can be a useful tool for programmers to add notes or explanations to their code without affecting its functionality. Example
Comments in Python don't always have to be an explanation of the code. They can also serve as a way to prevent code from being executed. Example
Multiline CommentsIt is crucial to understand that Python does not have a designated syntax for multiline comments. Nevertheless, you can accomplish this by utilizing a "#" for each line. Example
In Python, if you fail to assign a multiline comment to a variable, the code will be read but ultimately ignored.
|
What's Your Reaction?
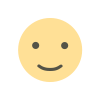
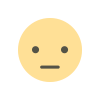
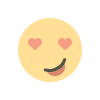
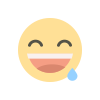
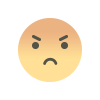
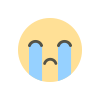
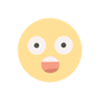