Arrays in Python: Unlocking the Secrets of Data Organization
Explore the world of Python arrays in our comprehensive guide. Master efficient data handling techniques, boost performance, and elevate your coding skills today.
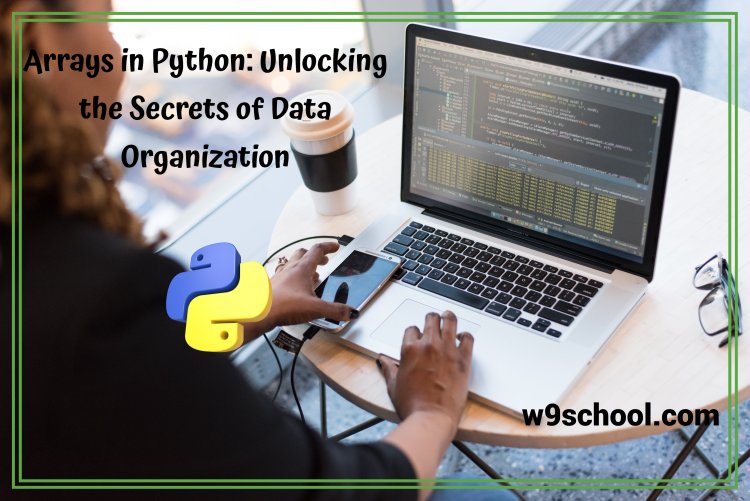
Python Arrays |
Python arrays are an immensely helpful way of storing multiple values of the same data type at once, simplifying program operations by decreasing traversal times for datasets to retrieve needed values, as well as offering string operations like slicing and concatenation. Python arrays are collections of items that can be accessed using a unique index value, starting at zero and progressing up to the last element in the array. This value can be changed at any time to add or remove items from the array, plus it supports all of the same functions that lists do. To create an array in Python, import the array module at the beginning of your script file. From there, all available array functions will become accessible. Python arrays are mutable, which means that their contents can be changed at any time; however, you must take care not to alter individual item's data types, as this could lead to errors. Additionally, an array requires all elements to have the same data type, making combining data of various types more complicated than it needs to be. To mitigate this issue and achieve your goal of merging different kinds of information into one collection of data more easily, it may be better to construct your array as a list first and convert it later into an array. When adding new elements to an array, using the insert() function will shift existing items right in order to make space for your new additions. For end-of-array additions, append() functions can also be used. Python arrays are most often used to store integers; however, they can also be used for any kind of data storage imaginable, including the names of students or employees stored as an array. When accessing this data with an index like [student name], an array would allow quick and efficient retrieval. Python stands apart from other programming languages by having just a single data type for arrays, making them far easier to manipulate using all standard string operations (slicing and concatenation). Furthermore, you can even nest multiple arrays within Python's handy capabilities! Python arrays can also be used for performing mathematical operations like division and multiplication, which makes them particularly helpful when programs require lots of input. Finally, their easy creation and manipulation make Python arrays one of the most widely used data structures in programming. |
Creating Python Arrays |
Note: Python does not provide built-in support for arrays However, Python lists are a substitute. |
Arrays |
NOTE: This page shows you how to utilize LISTS as ARRAYS However, in order to use arrays in Python you'll need to add a library such as NumPy Library. |
Arrays can be used to store several values in a single variable: Create an array of car names:
What is an Array?The term "array" refers to a specific variable that can store several values the same time. If you've got a checklist of things (a list of the names of cars such as) then storing them in a single variable could appear like this:
What if, however, you're looking to shuffle through the cars in order to locate one that is specific? What if you had not just three vehicles and 300? The answer is array! An array could contain a variety of different values in a single name. You can access the array's contents through one of the numbers. |
Access the Elements of an Array |
It is possible to refer to an element of the array through the Index number. Example Find an array value for the item in the initial range:
Example Alter the array's value:
The Length of an ArrayUtilize using the Example The number of components contained in the
|
Notice: The length of an array is always greater than the index of the highest array. |
Looping Array Elements |
You can make use of this Example Print every item of the
|
Adding Array Elements |
You can utilize an Example Add another element to your
|
Removing Array Elements |
You can employ pop() to use the Example Eliminate the second component from the
You may also employ remove() to use the Example Remove the element with"Volvo" as its value "Volvo":
NOTE: The list's |
Array Methods |
Python Arrays Vs Python Lists |
Arrays are ideal for large sets of homogenous items that need quick operations performed on them while being efficient with memory usage and storage needs. But for complex math or heterogeneous data sets, lists may be better options; to select the optimal data structure for your needs, it is crucial that you understand the differences between Python arrays and lists. Python arrays and lists both allow for the storage of an ordered, easily modifiable sequence of values in an index-like structure. Both use square brackets as building blocks, using similar syntax; their main differences between themselves are that a list can nest while an array cannot. In addition, lists allow different objects of various types to be stored, while an array requires all its elements to be of one type only. Python arrays stand out against their list counterparts by being faster and more efficient when accessing or editing data within them. Since their elements are stored continuously in memory, accessing an array can typically happen quickly, as computers can quickly look up an index number to locate its memory location.
One disadvantage of Python arrays is their complexity and memory consumption; computers must keep track of every individual item contained within an array, leading to additional pointer use in order to maintain this system. If you want to change the value of an array element, if necessary, a whole array must be copied over and updated with its updated value. This can be particularly inefficient when dealing with large arrays that change frequently. Reindexing all elements within an array is another consideration if an array is modified, which may take considerable time depending on its size and complexity. If this becomes a problem for large arrays, reindexing all of their elements could take several days before completion. Finally, Python arrays require more space than lists due to the need to construct individual Python objects for every data-type entry. While this should not be an issue with small arrays, this limitation can become restrictive when trying to store heterogeneous data or perform complex math functions on them. Luckily, packages like Numpy provide arrays with additional flexibility. |
Conclusion |
An array is a data structure used to store multiple values that can be accessed using their index numbers, much like a list. An array has the additional feature of being dynamic: you can add or remove elements as necessary from it. Python provides two methods for creating empty arrays: array (data type, value list) or fromlist() or frombytes(), which create an initial placeholder array you can fill later. Python arrays can store more than integers; you can store strings and floats as well, making histogram arrays easy for visualizing data points from various bins. Indexing allows you to access individual elements in a Python array using their unique identifier, or index number. Python index numbers always begin at zero, whereas most programming languages start counting from 1. To access an individual item from an array, first write its name followed by square brackets ([]) before providing its index number within those brackets. As well as indexing methods, Python arrays also support slice operations that allow accessing individual items through [Start Index:] or [-Index:]. Finally, to print all elements within an array, use print (python array). To delete an element from a Python array, use its built-in remove() method with its parameter value to be removed from it. Iterators can be used to loop through and delete each of its elements one at a time. Python offers you several built-in methods for inserting new values into an existing array. One such is its insert() method, which takes as its argument the list of elements you wish to add, inserts them at the end, and calls extend() in one go if multiple values need adding simultaneously. If you want to change the shape of an array without altering its data, the reshape() function provides an easy solution. Unfortunately, however, this only works with arrays that use one-dimensional layout; two-dimensional arrays require more advanced tools, such as transpose(), which offers similar flexibility as reshape() but more easily fits your needs. Use the join() function to easily combine two arrays of similar data types. Similar to SQL databases, this function returns an output containing all elements combined from both arrays, making this function ideal if you need to perform calculations on two large datasets simultaneously. |
For more information, Please visit Home
What's Your Reaction?
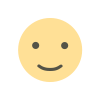
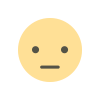
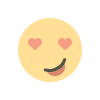
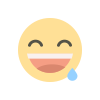
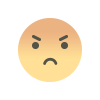
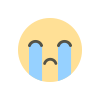
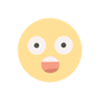