Python Math Secrets for Efficient Programming : Numeric Nirvana
Explore the depths of Python Math in this comprehensive guide. From fundamental concepts to advanced techniques, empower your code with precision and elegance.
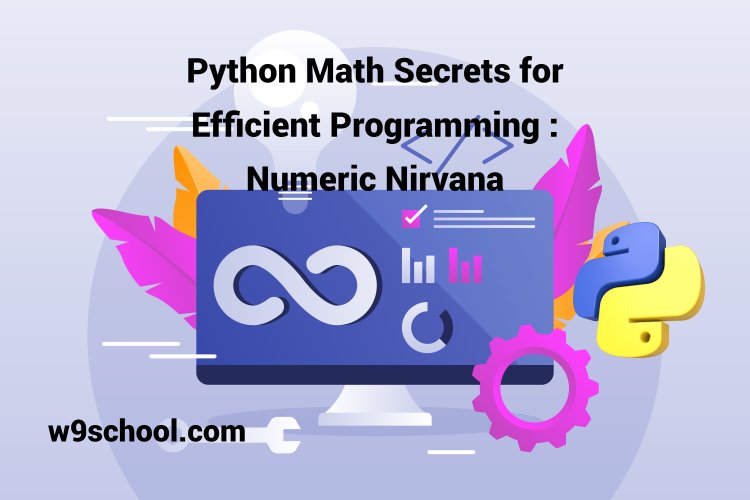
The Math Module in Python |
Python is an accessible programming language with many mathematical calculation functions and modules such as Math that make life easier when writing programs in Python. These modules make adding additional functionality easier than ever! The floor function returns the largest integer less than or equal to x. Meanwhile, ceil approximates this number as being greater than or equal to it. ConstantsPython modules are an invaluable resource that can help your program function more smoothly. They contain pre-written code items such as objects, functions and constants not available within the base language itself - for instance the math module offers common mathematical functions. Constants are values expected to remain constant over the lifetime of your code. Python lacks a dedicated syntax for constant definition, so when referring to them it is essential that a standard naming convention be followed when writing code. Capital letters should be used when writing the name of constants with an underscore between words as part of this convention. A straightforward approach to managing constants in Python is defining them at the same time as their use; this makes managing them simpler, as their definition will occur nearer the top of each module that contains their related code.
PiPython is an incredibly flexible programming language capable of performing mathematical calculations quickly. When working with large numbers or complex formulae, understanding its mathematics module and how it should be integrated into your code are especially crucial. The math module gives access to several mathematical functions defined by C standard, however these cannot be used with complex numbers; please see cmath module if that's needed instead. The math module contains various functions, such as ceil() and floor(). Ceil() approximates a value to its lowest integer while floor() returns the largest integer less than or equal to a float - these features can help calculate area for complex shapes such as circles. TauModules are an indispensable element of Python programs, enabling you to import objects, functions and constants without writing them from scratch. One such module is math which enables mathematical operations. Python provides several built-in mathematical functions that you can utilize for various tasks, including performing arithmetic, logarithmic and trigonometric calculations. Furthermore, its Math module also offers representation functions like ceil() and floor() that make use of this functionality even simpler. The Math module is a standard component of Python and should always be available; to access it, import it via import math statement. Unfortunately, however, it does not support complex numbers; to do this you must utilize cmath module instead. Square RootSquare root is an operation used to convert numbers to their squares, often used in geometry and quadratic equations. Python's math module offers an efficient square root function which quickly and accurately produces this information. Python's sqrt() function converts any number to float format, while you can also use pow() or ** as alternatives to achieve similar results. However, these methods are less convenient than Python's math module's sqrt() function, which works with all numbers and returns a float. Unfortunately it does not work with negative numbers - any attempts at taking their square root would result in a ValueError exception since square roots must only ever be positive numbers. SinePython contains many predefined mathematical functions which are very helpful when performing advanced calculations. Furthermore, there are constants which you can utilize within your code. The ceil() function approximates a given value to the nearest integer greater than or equal to it, returning a float as an answer. Meanwhile, floor() returns the largest integer less than or equal to any given number as an integral. The sqrt() function returns the square root of any number while exp() returns its power of expansion. You can also calculate an exponential of any value by using pow(); in this code example it's also possible to calculate pow() with pow() being expanded until reaching desired values and print out. Finally a summation is calculated and printed out. |
Creating Python Math Module |
Python includes a range of math functions built-in, including a comprehensive math module that lets you perform math-related tasks with numbers. Built-in Math FunctionsIt is possible to use the Example
The Abs() function returns the absolute (positive) value of the number specified: Example
Its Example Restore that value by multiplying it with 3 (same like 4*4*4):
|
The Math Module |
Python includes an integrated module known as To utilize it, you have to install your
Once you have installed into the It is the Example
It is the Example
Math.pi constant, also known as the Example
|
Complete Math Modules |
In Math Module Reference, you will find a complete reference of all methods and constants that belong to the Math module. Python has a built-in module that you can use for mathematical tasks. The Math Methods
Math Constants
|
For more Information, Please visit Home
What's Your Reaction?
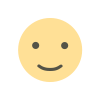
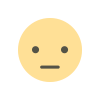
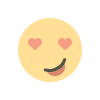
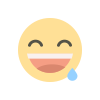
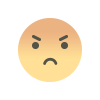
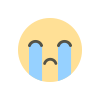
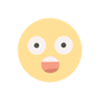