Python Polymorphism Demystified: Crafting Flexible Programs
Dive into the world of Python polymorphism and discover the art of crafting flexible, dynamic code. Unleash the power of versatility in your programming journey.
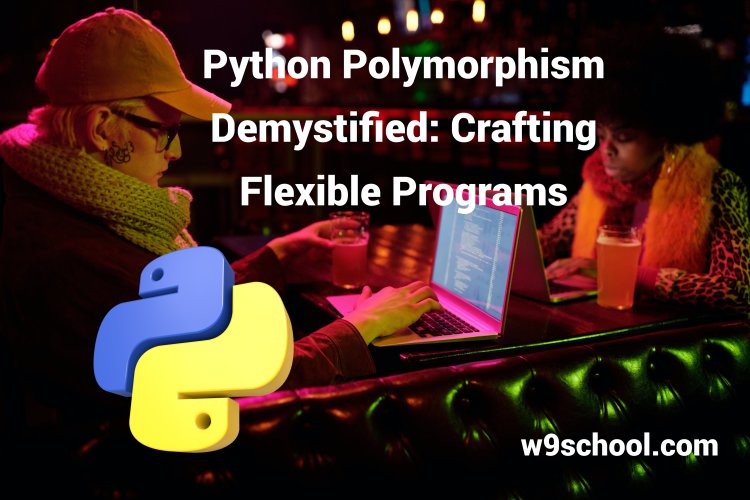
Python Polymorphism |
The word "polymorphism" means "many forms", and in programming it refers to methods/functions/operators with the same name that can be executed on many objects or classes. |
The Importance of Polymorphism in Python Programming |
Polymorphism in Python fosters modularity by eliminating unnecessary duplication in code, while making debugging simpler by making it easier to locate and resolve bugs. Python offers many different strategies for achieving polymorphism. While inheritance is usually the preferred approach, you can also utilize function and class methods as illustrated by the example of the + operator above. InheritanceInheritance is an object-oriented design technique that enables one class to share attributes and methods with another, which reduces redundant code while increasing reusability. The class that inherits is known as the parent (base class) while those being inherited are known as children or derived classes. A derived class can override inherited methods from its parent class to alter how they work for it. When Python calls a method on such an entity, it checks whether any modifications were made and, if so, executes its new version instead. Multiple inheritances can become complex quickly when one child class inherits from multiple different classes - known as the diamond problem. Python offers a solution called mixins to alleviate this complexity: mixins are classes which provide methods to other classes but do not act as base classes, making adding functionality easy without increasing complexity of an application. CompositionPython programming supports polymorphism as a concept that enables one function to take different forms depending on its circumstances, providing more reusable and adaptable code for developers. Python supports this idea through function overloading, duck typing, and abstract classes—three ways of supporting this principle. Another form of polymorphism can be seen when using the + operator to add values of different types. For instance, this operator works equally well when adding two integers as it does when adding two floats or strings—known as coercion polymorphism. Python also facilitates polymorphism through inheritance and method overriding techniques, which allow base classes with methods that can be overridden by derived classes for customization by calling their overridden version of that same base class's method; when this happens, Python evaluates the type of object called and executes appropriate code accordingly. Method OverloadingMethod overloading is a form of polymorphism that allows classes to have multiple methods with identical names but different input parameters, providing greater flexibility and versatility in Python code. Furthermore, method overloading helps programmers write cleaner code by grouping related functionalities into cohesive functional blocks. This concept is closely tied to inheritance: subclasses can override methods in their parent class by providing specific implementations of those methods; when called from within a derived class, Python interpreter selects which version was implemented by that subclass. The "+" operator provides an excellent example of polymorphism at work. This operator can be used to add various integer, float, and string types together and also take on different forms depending on context—an attribute that showcases Python's flexibility and object-oriented programming power in real-world situations where software systems need to adapt and change with user needs. Policy-Based DesignPolicy-based design is an object-oriented programming technique in which classes' behaviors are implemented using policies instead of directly coding. This approach helps eliminate unnecessary if statements and improve code clarity while simultaneously decreasing maintenance costs as the policies can be configured during compile time. Python offers policy-driven design through function overloading and method overriding, which allow functions to use objects of a particular class in various ways and promote flexible and extensible code development. Python supports polymorphism through duck typing and operator overloading, which enables its addition function to function differently depending on what kind of data it receives. If the data comes in as integers, the addition function adds them together, while otherwise it concatenates them based on duck typing or operator overloading rules, allowing reuse of one function name across various types. This concept allows the reuse of an int function name across many data types without changing it to reuse it as needed. |
Function Polymorphism |
A good illustration of an Python function that could be applied to different object types is len() function. StringStrings Example
TupleFor tuples, Example
DictionaryFor dictionary databases, Example
|
Class Polymorphism |
Polymorphism is commonly employed often in Class methods, in which there are multiple classes that share the same name. As an example, let's say there are three classes: Example Different classes that use the same approach:
Take a look at the for loop that is at the close. Due to polymorphism, we are able to use the same technique for the three classes. |
Inheritance Class Polymorphism |
What happens to classes that have children classes that have identical names? Do we have the option of using polymorphism in those classes? Yes. If we follow the above example and create an object class called Vehicle, and create the Car, Boat and and Plane Child classes of Vehicle these classes inherit the methods used by Vehicle but they are able to modify them to: Example Make a class titled Vehicle. You can make a Vehicles, such as a Boat or Car. or Plane children classes of Vehicle:
The child classes inherit the methods and properties of the parent class. In the above example, you will notice that the class Car is not empty, however it inherits model, brand as well as the ability to move() of Vehicle. It is also important to note that the Boat Classes and the Plane inherit model, brand, as well as model() of Vehicle however, they both use their move() method. Since polymorphism is a feature, we can apply the same procedure to all classes. |
Conclusion |
What is Polymorphism in Python?Polymorphism is an essential concept in object-oriented programming as it allows one action to be executed multiple ways and allows developers to build flexible and scalable programs. Polymorphism in Python can be achieved using inheritance and function overloading; this allows classes to have methods with identical names but Python will determine which version should be called depending on which object type it encounters based on inheritance and function overloading; this practice is known as method overloading and contributes significantly to making Python so flexible. Polymorphism in programming can help reduce code duplication and maintainability issues while making debugging much simpler, making bugs much simpler to identify and address. Furthermore, using this strategy enables flexible applications that are able to adapt easily in response to changes in user behavior without needing major code revisions. Polymorphism is an essential principle of object-oriented programming. It refers to an object's ability to take various forms, which enables it to adapt more readily to its environment and users' needs. Python makes this possible with polymorphic functions and objects - something which has made the language so popular among developers. Len() is an example of polymorphic function; it can be applied to various data types like strings, lists and dictionaries while still returning its same length value. This is similar to how adding methods (or + operators) work within classes which handles integer addition, floating point addition or bignum addition - these all return their respective length values regardless of which data type the addition occurs on. Inheritance and method overriding are two of the primary forms of polymorphism in Python. Inheritance allows child classes to override methods from their parent class; this can be useful when the business logic doesn't match those defined in a base class, thus providing a new version of that method which can then be passed back onto the Python interpreter for processing. Method overriding is an essential aspect of Python that allows you to redefine a function with the same name that has already been defined within another subclass. Python will evaluate how many arguments have been passed into a function before calling out its correct version of it. Animal classes contain methods called make_sound() that print generic messages; Cat and Dog classes override this method so it will output different responses depending on which animal type it's applied to - thus turning Animal into a polymorphic object. |
For more informative blogs, please visit Home
What's Your Reaction?
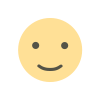
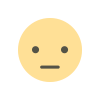
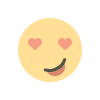
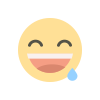
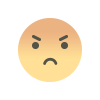
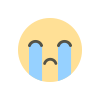
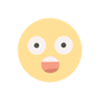