Elevate Code Mastery: C++ Arrays Unleashed! - w9school
Optimize your code with dynamic C++ arrays! Unlock trends in programming excellence. Master arrays for efficient, high-performance solutions. Dive in now!
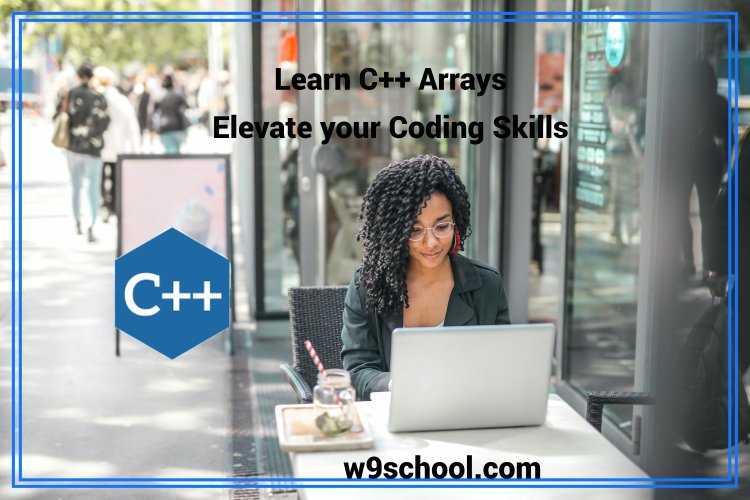
What Are C++ Arrays? |
C++ arrays are data structures which store values of one type consecutively in memory locations and allow their access using a unique index, making them suitable for working with large sets of homogenous data such as scores or temperatures. An array is also faster than declaring multiple variables of similar type (for instance five integers) individually and accessing each one individually as it requires less code. At its declaration, an array has a fixed size that is specified at that moment; this makes it perfect when you know exactly how many elements will be necessary and want to enforce that limit. Furthermore, using LIFO technology similar to that found in std::vector makes working with such structures faster than working with stacks. Accessing an array is achieved by adding an index number to its base location; for instance, in an array with 8 elements it may be reached by indexing to [0]. Indexing past the end of an array could result in garbage data or even cause it to crash due to C's lack of built-in range checking on array accesses; so take caution when indexing. As another downside of arrays, they lose their knowledge about their size when passed between functions, leading to something known as array decay. Therefore, vector data types like std::vector are usually preferred when creating collections data types. Arrays are one of the core data structures in computer programming. They enable programmers to group multiple elements of a single type under a single variable name, serving as the foundation of many important algorithms and other structures. arrays are relatively straightforward data types to work with compared to structures or pointers which often require more care when being utilized correctly, arrays are relatively effortless in their application. Declaring several variables of one type under one variable name and accessing individual elements via their index number makes using arrays an effortless experience. It can store both primitive data types (ints, floats, doubles etc) as well as more derived types (lists structures even unions). When declaring an array, its compiler reserves an amount of memory space for its members and records this information in its memory mapping file - similar to an address book for computers. The compiler keeps track of where members of an array start and end in terms of time spent within an array. When searching, starting at its last member it logically moves forward until reaching a member in need. As you might expect, arrays are extremely helpful when your program contains a large volume of similar data which would otherwise require multiple variables to store it. Furthermore, arrays allow for simplified programming code as you can access all the variables with a single loop (such as for loop) rather than individual loops for each variable in an array. There are many advantages of arrays, such as memory efficiency and sequential access. Memory efficiency comes from their use of contiguous memory allocation, making them more cost-efficient than linked data structures when working with large datasets or time-sensitive tasks; similarly, sequential access allows easier processing and manipulation while reducing round trips needed to store and retrieve the data - these advantages may lead to improved performance in certain instances. Initialization: Arrays can be initialized immediately at declaration by providing an initializer list enclosed within curly braces - known as direct initialization. You can also initialize an array by creating its members one at a time through an array loop such as for loop, with each member separated from its neighboring members by commas; to find the 3rd member of an array containing 5 members you would specify an initializer list as marks[0][2]. Certain languages, like BASIC and JAVA, require care when accessing arrays. As these languages do not perform range checking, if you access an array element past its declared size then your program could encounter issues which are difficult to debug and could even stop running altogether resulting in unpredictable results. |
C++ Arrays |
Arrays can be used to store a variety of values in the same variable, instead of declaring distinct variable for every value. For declaring an array specify the type of variable, then specify its name, followed with in brackets and then specify its number of components it will be able to store:
Now we have declared a variable which holds four strings. To add values to it you can make use of an array literal. Place the contents in a comma-separated list inside curly braces:
To make an array consisting of three numbers you can write:
Access the Elements of an ArrayYou can access any array component by referring it to the index number in round brackets This statement is a reference to the significance in this initial part in automobiles: Example
|
Notice: Array indexes start with zero: [0] is one of the elements. The [1] element is the next element, and so on. |
Change an Array ElementTo alter the value of an element, you must refer to an index:
Example
|
C++ Arrays and Loops |
Loop Through an ArrayIt is possible to loop array elements by using for loop. The following example shows all the elements of the cars array: Example
This example shows an index for each element, along with the value of each element: Example
This example demonstrates the way to cycle through a set of integers: Example
The foreach Loop
There is an additional " for-each loop" (introduced in C++ version 11 (2011) that is exclusively used to loop through elements of an array: Syntax
This example shows all the elements of an array, employing an " for-each loop": Example
|
C++ Omit Array Size |
Omit Array SizeWhen using C++, you do not need to define how big the array will be. The compiler is intelligent enough to estimate its size based upon the number of values that are inserted:
The above example is equivalent to:
The last option is considered to be "good practice", because it can reduce the likelihood of making mistakes in your program. Omit Elements on DeclarationYou can also declare an array without indicating the elements in the declaration and later add them: Example
|
C++ Array Size |
Get the Size of an ArrayTo determine the dimensions of arrays you can utilize sizeof() operator: Example
Why does the result show It's due to it is because the You learnt through chapter section on data types the fact that int type is typically 4 bytes. To determine the number of elements an array contains you need split the amount of space by the type of data it holds: Example
Loop Through an array using sizeof()The arrays and Loops Chapter, we have written that the dimensions of an array within the loop conditions ( But, applying an Instead of writing:
It is more effective to write: Example
Be aware that with C++ version 11 (2011) you are able to also make use of"for-each" loops. "for-each" loop": Example
|
It is helpful to understand the various ways to loop through an array since you will likely run into them all in different applications. |
C++ Multi-Dimensional Arrays |
Multi-Dimensional ArraysA multi-dimensional array is a collection of arrays. In order to declare a multi-dimensional array specify the type of variable, then specify that the names of the array, followed by square brackets that specify the number of elements that the main array contains, then an additional set of brackets, which specifies the number of elements that sub-arrays contain:
Like normal arrays, it is possible to insert values by using an array literal. It's an comma-separated, comma-separated braces. When you have a multidimensional array every element of the literal array is one literal array.
Each square bracket inside an array declaration is an additional dimension to an array. An array such as the one shown above is believed to contain two dimensions. Arrays can be created with the number you want. The larger the array's dimensions the more complex the code is. The following array is composed of three dimensions:
Access the Elements of a Multi-Dimensional ArrayTo access an element within an array that is multi-dimensional, you must specify an index number for every dimension of the array. This statement retrieves an element's value that is located in the first row (0) and the third column (2) of the alphabet array. Example
|
Keep in mind the following: Array indexes start with zero and [0] is the element that is first. "1" is the 2nd element, and so on. |
Change Elements in a Multi-Dimensional ArrayTo alter the element's worth use an element's index in all dimension: Example
Loop Through a Multi-Dimensional ArrayIn order to loop through multidimensional arrays you'll need a loop for each array's dimensions. In the following sample, you can output every element within the alphabet array: Example
This illustration shows the process of looping through three dimensions Example
Why Multi-Dimensional Arrays?Multi-dimensional arrays excel for representing grids. This example illustrates a real-world application for multi-dimensional arrays. In the following example, we employ a multi-dimensional array to represent a game called Battleship: Example
|
Summary |
An array is a grouping of data belonging to the same data type and category stored in contiguous memory locations. Index numbers start from 0, making accessing them simple. They're used instead of declaring multiple variables for similar types, saving both time and effort when working with large volumes of information, plus making your code easier to read. An array can be created using any fundamental data type such as int, float, double and char as well as user-defined types like structures or classes. When declaring an array, its number of elements is specified at its creation; its size cannot be changed later. When passed as pointers to functions when called, arrays lose any knowledge about their size due to "Array Decay", potentially leading to errors and memory leakage. Arrays offer many advantages, the primary one being accessing elements in a data set in one loop using indexes. This makes data processing simpler while improving efficiency; plus they're highly scalable and work well with legacy code or APIs. Arrays offer another great benefit in that initialization of their elements takes place at the time of declaration. We give an array a name and specify its size with numbers within square brackets []. Unlike other storage types which allow multiple variables per data type value to be entered at once, arrays allow only a limited number of values at one time that are determined during declaration.
|
For more information, please visit Our website .
What's Your Reaction?
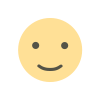
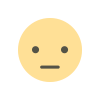
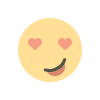
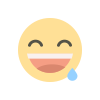
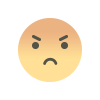
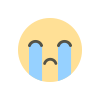
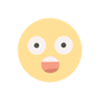