Elevate Codecraft: C++ References & Pointers Mastery - w9school
Dive into the world of C++ references and pointers. Unlock precision in code manipulation. Elevate your programming expertise for efficiency and innovation. Start learning now!
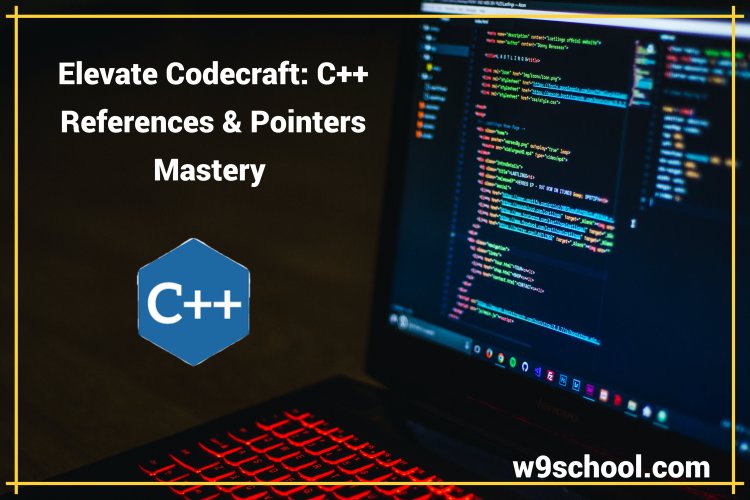
What is a C++ Reference? |
Referencing variables is similar to creating an alias; you can refer to it via its address or name; however, unlike pointers, NULL cannot serve as the name for a reference name. Pointers allow computer programs to indirectly access certain data stored within memory or on other storage devices, such as variable values or records. To do this, indirection operators such as the dereferencing operator * must be used. Referencing variables is an integral component of C++ programming that provides you with an alternative to pointers. While similar, references offer some distinct advantages over their pointer counterparts; for instance, they can be passed more securely to functions than pointers can, and they also reduce copying when passing variables into functions. References can also be used to change the value of local variables in one function by calling a function that takes a reference to them rather than copying over their value into new variables, which could potentially be more costly and time consuming. This feature is known as pass-by-reference. References can be initialized with either lvalues or rvalues, which allows for functions or overloads of functions which accept only rvalue arguments - making these much safer than functions which accept only lvalue arguments. Rvalue references can also be used with the std::move function to give permission for an rvalue argument to access resources that would normally have been unavailable to it. Rvalue references are a welcome addition to the language, as they allow you to write more robust code using move constructors, assignment operators, and other functions that manipulate moveable values more safely than before. Furthermore, templates with this capability allow them to detect whether an argument is an rvalue or an lvalue and make templating decisions accordingly. One possible exception for when references might not be valid is when the variable they point to changes value while they still refer to it. When trying to access that memory location, however, a segmentation fault or access violation could occur; in practice this shouldn't be an issue since references typically keep an old copy of original value and address of their target variable for use as references themselves. |
Creating References |
Reference variables are an "reference" to an existing variable. It is constructed using the
Then, we can utilize any of the variables named Example
|
C++ Memory Address |
Memory AddressIn the example on the earlier page In the example from the previous page, When the variable is created in C++, a memory address is assigned to the variable. When the variable assigns a value the variable, it's kept in that memory. In order to access the variable, utilize the Example
|
NOTE: The memory address is in hexadecimal format (0x ..). It is possible to have the exact same result using your program. |
What is the reason to have what memory addresses are?references as well as points (which you'll learn about in the following chapter) are essential when working in C++, because they enable you to alter the data stored in the memory of the computer which can be used to manipulate the memory of the computer which could decrease the code and increase efficiency. Two of these features are just one of the features that makes C++ stand out from other programming languages such as Python as well as Java. |
C++ Pointers |
The Basics of C++ PointersC++ programmers have access to an extremely powerful tool when they employ pointers: data manipulation in memory is directly accessible, making complex data structures possible and speedier access. They also help optimize performance by decreasing memory lookup times required when accessing data, but when misused can become confusing and error-prone. In this article, we will investigate the fundamentals of C++ pointers and provide examples that demonstrate their power and versatility. Additionally, we will also address common mistakes made while using pointers, along with ways to avoid them. As soon as a variable is declared, its memory requirements are allocated a location within memory (its address). This address is unique for each variable and can be used to access its cells directly. Most C++ programs leave this task up to the operating system, however if you wish to have more direct control over how your memory is allocated and deallocated using smart pointers you may do so yourself. Smart pointers are an innovative C++ feature that helps avoid common pointer errors by managing memory usage for their underlying objects. Initialized with make_shared or make_unique_ptr, smart pointers like these manage their own objects when out of scope and delete themselves automatically from memory upon exiting scope. Pointers in C++ are variables that store the addresses of objects. To access it, the dereference operator takes this address and returns what value the pointer points to; you can perform mathematical operations with it like adding and subtracting using regular integer types, although pointers behave slightly differently due to their individual data types' sizes. Addition or subtraction are both capable of changing the value that the pointer points to, subject to the constraints imposed by its data type (int or char). However, subtraction from a non-canonical address would generate a general protection fault from its compiler. Use an array index as another means of accessing the value pointed by a pointer. For instance, the following code uses this approach when looping through an array and printing each element - using first an index while secondly using pointers - while printing. Keep in mind when declaring pointers that when declaring them an asterisk should always precede their name as this signifies they require dereference in order to access its value. Inserting spaces around an asterisk won't affect its meaning but could cause unnecessary confusion when reading code. |
Creating PointersIn the previous chapter that you can find an address of memory for a variable using the Example
Pointers, however, are a type of variable that pointer however is a variable that is able to store the memory address in it's value. A pointer variable is the kind of data (like Example
Example explained Create a pointer variable using the name ptr. It refers to a string variable through the use of an asterisk symbol * (string* ptr). Make sure that the kind of the pointer must be the same as the type of variable you're working on. Utilize the Then, |
TIP: There are three methods for declaring pointer variables; however, the first method is the preferred one:
|
C++ DereferenceGet Memory Address and ValueIn the example of the earlier page, we utilized the pointer variable in order to find an address for memory of the variable (used along with the Example
|
It is important to note that the
|
C++ Modify PointersModify the Pointer ValueYou can also alter the value of the pointer. Be aware that this can affect values of the initial variable: Example
|
Pointers Vs References in C++ |
Pointers and references both provide indirect access to data, but their differences make knowing when and why to use each a vital aspect of good programming, often saving from serious bugs. Deciding when and why to use one is often determined by what task needs doing; many C++ programmers develop some sense of intuition regarding which pointer or reference would work better given a particular situation. Think about a function that takes in variables by reference and passes them back out again; technically speaking, this gives the same result as passing in pointers directly, but its code becomes much more complex when passing variables by reference, and its use can prevent certain important features of C++, such as garbage collection, from working properly. An important distinction between pointers and references lies in their identities: while pointers can be obtained with unary & and require their own space on the stack, references simply require the space already taken up by variables bound to them on the stack. Due to this difference, pointers enable more arithmetical operations, while such operations cannot be conducted on references. Pointers can also be assigned to different variables, whereas references cannot. This difference is essential when creating linked lists and trees, as it impacts implementation strategies. As soon as a pointer is altered, the compiler automatically changes the lifespan of any temporary objects that it points at, making a noticeable impact on performance and helping prevent memory leakage. Pointers should only be used with variables of the same type. Their syntax differs slightly, with pointers having an asterisk symbol for declaration and references using a forward arrow symbol ->; however, this distinction is just syntactic sugar; at its core, a reference is just another name for a variable, while your compiler keeps track of every variable by associating its name with a unique memory address; therefore, it is impossible for pointers to point towards references or null pointers. |
For more information, Please visit Home
What's Your Reaction?
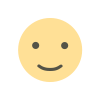
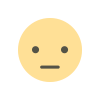
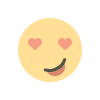
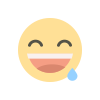
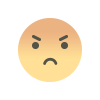
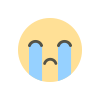
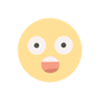