C++ Classes Unleashed: OOP Excellence For Beginners to Masters - w9school
Dive into C++ classes for unparalleled Object-Oriented Programming. Unleash coding excellence, master encapsulation, and elevate your software design skills. Explore the essence of OOP now!
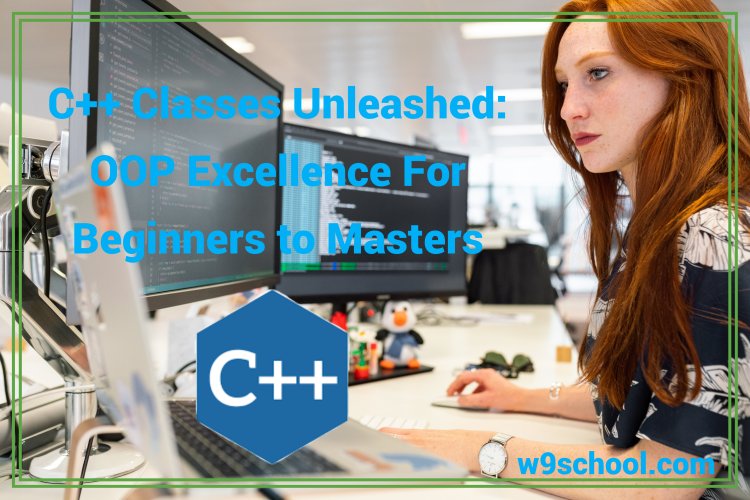
C++ OOP |
C++: What is OOP? OOP is an acronym for Object-Oriented Programing. Procedural programming involves writing functions or procedures which perform operations on data, whereas object-oriented programming is the creation of objects that can contain functions and data. Object-oriented programming has many benefits over procedural programs.
Tips: The "Don't Repeat Yourself" (DRY) principle is to reduce the repeating of codes. You must remove code that is common to the application, then place them in a single location and reuse them instead repeating the same code. C++: What are Classes and Objects? Classification and object are two major elements of object-oriented programming. Take a look at the image below to discover the differences between objects and classes:
Another illustration:
A class is an object template, and an object is a subset of the class. When individuals are made they inherit all variables and functions of the class. You'll learn details regarding subjects and classes during the chapter. |
C++ Classes and Objects |
C++ Classes/ObjectsC++ is an object-oriented programming language. All of the things in C++ is associated with objects and classes as well as their properties and techniques. For instance, in actual life, a car can be described as the definition of an objects. It has characteristics like the color and weight, and techniques that are used to drive it, for example and brake. Methods and attributes are essentially the same as variables that are essentially variables and functions that belong to the classes. They are usually described as "class members". The term "class" refers to a type of data that can be defined by the user that we can utilize in our programs, and it functions in the role of an object constructor or as a "blueprint" for creating objects. Create a ClassFor creating a class make use of to create a class, use the Example Create a class titled "
Example explained
Create an ObjectWhen using C++, an object is created using an existing class. We already have created a class called To create an instance of To access the attributes of the class ( Example Create an object named "
Multiple ObjectsIt is possible to create multiple objects from the same class: Example
|
C++ Class Methods |
Class MethodsThe methods are functions which belong in the same class. It is possible to define a function that belong to a class:
In the next example, it is possible to define a method in the class, and give it the name " NOTE: You access methods similar to attributes. You do this by creating an object in the class applying the syntax dot ( Inside Example
In order to create a new function that is not part of the definition of a class it is necessary to declare it within the class, and afterwards define it out the class. This is accomplished by defining your class's name following by the scope resolution Outside Example
ParametersYou can also add parameters Example
|
C++ Constructors |
ConstructorsThe constructor method in C++ is a specific technique to be automatically invoked when an object in an existing class is made. To build a constructor apply exactly the same class name then use in parentheses Example
|
Note: The constructor has the same name as the class, and it remains |
Constructor ParametersConstructors also have the ability to take parameters (just as regular functions) and can be beneficial for creating initial values for attributes. The following classes include Example
Like functions, constructors can be declared outside of the class. First declare the constructor inside the class. Then, define it outside the class by defining that the title of the class then an appropriate scope resolution Example
|
C++ Access Specifiers |
Access SpecifiersAs of now, you're already familiar with that Example
A What do we do if we want our members to be kept private and away from view? Within C++, there are three access specifiers in C++:
In the following example, we illustrate the difference between Example
If you attempt to log in as the private members, the following error is triggered:
|
Note: It is possible to gain access to individuals who are private in a particular class by using public methods within that same class. Check out in the following chapter ( Encapsulation) on how you can do this. Tips: It is considered an excellent method to mark your class's attributes confidential (as regularly as are able to). This can reduce the chances of you (or other people) to make mistakes in the code. This is also the primary component to this concept of the Encapsulation concept that you will be able to discover more about during the subsequent chapter. |
Note: By default, the members of a class can be Example
|
C++ Encapsulation |
EncapsulationThe purpose the purpose of Encapsulation is to ensure the "sensitive" data is hidden from the view of the user. In order to achieve this, you have to declare the class variables and attributes as Access Private MembersTo gain access to an attribute that is private, you must make use of the private "get" and "set" methods: Example
Example explained This This public Public In Why Encapsulation?
|
The Importance of Classes in C++ Programming |
C++ classes are the cornerstone of object-oriented programming languages like C#. Combining data and functions, they allow developers to build objects that can be used directly within code. A class can be seen as similar to a blueprint; its form and purpose being determined. Classes are sometimes also referred to as data structures. Classes provide your code with a much more modular structure, helping you write more efficient programs and make them more manageable. This is particularly helpful when working on large projects. C++ programming language supports various classes such as: enum classes, default constructors, static constructors and templated classes to make coding simpler and more manageable. Writing programs using an object-oriented style offers several benefits. These include increased performance, greater reusability and reduced bugs; as well as making the code simpler to understand and debug. An OO style often makes code easier to comprehend and debug; its main distinguishing feature being its ability to combine data and function into one entity - this makes c++ an OO programming language; class is its main concept behind this OO model and key to its success. C++ classes are defined with the keyword class followed by one or more access modifiers that define how other functions interact with it and access its data members. To maximize encapsulation and protect data members from being accessed outside the class, classes should typically be made private as much as possible. Accessing the member functions of a class can be accomplished using the dot operator (.). A class may contain public, protected, or private member functions; any function can only access them if declared as friends of that class or granted explicit access permission by it. Classes may include special member functions known as constructors and destructors that are invoked when class objects are created; destructors are then invoked when classes go out of scope or are deliberately deleted from existence. A class can contain both member functions and global methods, which are called by other functions - similar to C's void function - to call other functions within it. Normally, C++ compilers will attempt to inline global methods as much as possible for improved code performance. Layout of non-POD classes in memory isn't mandated by C++ standard; however, many compilers use single inheritance and concatenation of fields to represent parent class types and child class fields in memory. This helps achieve performance comparable to that of languages with built-in complex types; however, this method can lead to lots of duplicate code in a program and should therefore be limited as much as possible in terms of number and length of global functions used within one code base. |
For more information, Please visit Home
What's Your Reaction?
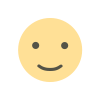
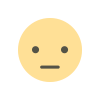
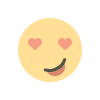
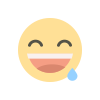
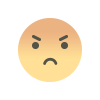
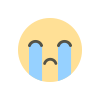
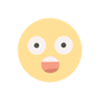