C++ Functions: Complete Guide Covering Every Aspect - w9school
C++ provides some pre-defined functions, such as main() , which is used to execute code. But you can also create your own functions to perform certain actions.
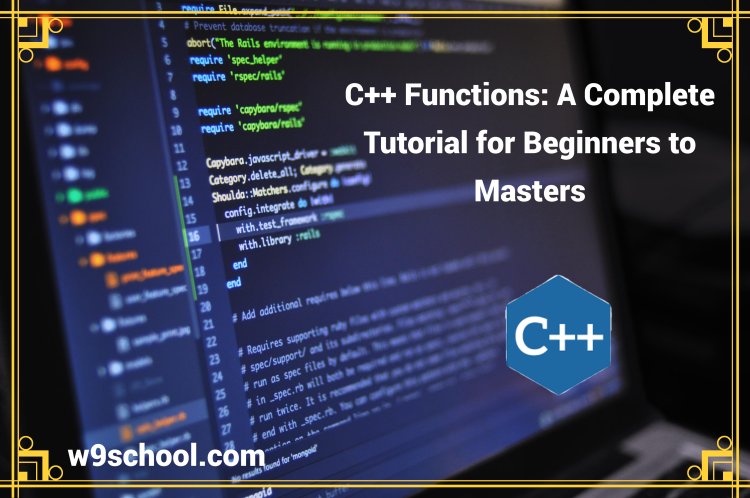
C++ Functions |
The term "function" refers to a section of code that runs only at the time it's invoked. You can input information, also known as parameters, to the function. Functions perform specific actions. They are crucial for the reuse of code. Create the code once and reuse it multiple times. |
How to Use C++ Functions |
Functions are an invaluable programming tool that enable you to structure your program into segments that accomplish one specific task, making the code simpler to read, edit, and debug. Functions can be used in programs for almost every purpose imaginable, from performing simple calculations and text generation, to allocating memory. To use functions effectively in your code, first declare them. After declaring, simply call them whenever required by calling them out in your code. There are two primary types of C++ functions: library and user-defined. Library functions allow you to draw upon premade functions for use in your program; user-defined ones enable more complex logic implementation and allow greater customization. To define a function, you must create a prototype and define its body. Furthermore, you should provide details regarding the function's name, return data type and parameter types; some functions do not return anything at all and in such instances must use void as the return type to indicate this fact. Functions must include a return statement in order to inform their calling programs of their results of execution. A function's return statement should always return an integer value that complies with its return data type (if any). When creating user-defined functions, it is recommended to give them names that clearly describe their task. This will make it easier for future programmers to comprehend what your function does. Also, keep the length of each function to seven lines or less for best results. You have two options for passing arguments to functions: reference or value. By default, C++ uses call-by-value which copies actual arguments into formal parameters; this ensures any changes made to function parameters don't change how arguments were passed in call-by-value method. Below is an example of a user-defined function to calculate the factorial of any given number, using three integer parameters x, y, and sum as input parameters and returning their sum as its return value. It can then be called from within your program's main() function for use. In our previous example, we read two input values from the user and then called the addFunction() function to calculate their sum. The result of the function was then printed out along with other text in our console. As we are writing our own programs, we need to decide how many functions and data will need to be stored within it. This will play an integral role in how much time we will spend writing the program as well as maintaining it after it has been written; so it is crucial that this decision is made as early in the process as possible. For instance, if creating an app with multiple users it would be prudent to break out functionality into separate functions. |
Create a Function |
C++ provides some pre-defined functions, like To construct (often called declaration) the function that is named, you must specify what the purpose of the program is followed by the parentheses (): Syntax
Example Explained
|
Call a Function |
Declared functions cannot be executed immediately. They're "saved for later use" and are executed later, after the time comes to call them. To invoke a function, compose the name of the function and then add two parentheses The following illustration Example Within
A function may be invoked multiple times: Example
|
Function Declaration and Definition |
C++ functions are a C++ function consist of two components:
|
Notice: If a user-defined function, like An error could occur: |
Example
|
It is however, possible to separate the declaration from functions definition to improve the efficiency of code. It is common to see C++ programs that contain function declarations over Example
|
C++ Function Parameters |
Parameters and ArgumentsInformation can be provided to functions via an parameter. Parameters function as variables within the function. Parameters are defined in the function's name within the parentheses. You can include any number of parameters you like, but break them up by a comma Syntax
The following example contains the function which takes the Example
|
If an parameter is given to the function, it's called"an" argument. In the above example the parameter fname is an argument that is a parameter, whereas Liam, Jenny and Anja are arguments. |
C++ Default Parameters |
Default Parameter ValueIt is also possible to use the default value for parameter with the use of an equals symbol ( If you call the function without an argument, it is using its default setting ("Norway"): Example
|
A parameter that is set to default value is commonly referred to as an "optional parameter". In the example above,
|
C++ Multiple Parameters |
Multiple ParametersIn your function, you have the ability to include as many parameters as you'd like: Example
|
Note that when you're dealing with several parameters, your function must contain exactly the same amount of parameters as are parameters. Additionally, the arguments should be sent in the same order. |
C++ The Return Keyword |
Return Values
Example
This example calculates the sum of a formula with 2 parameters: Example
You could also save the results in a variable Example
|
C++ Functions - Pass By Reference |
Pass By ReferenceIn the examples on the previous page, we made use of normal variables to pass parameters to functions. It is also possible to use an explicit references in the direction of your function. This is useful for when you want to alter the value of arguments: Example
|
C++ Pass Array to a Function |
Pass Arrays as Function ParametersIt is also possible to send arrays to an application: Example
|
Example Explained This function ( In the event that this function gets invoked within Note that when you call the function, you only need to use the name of the array when passing it as an argument |
C++ Function Overloading |
Function OverloadingWith overloading functions Multiple functions may be named the same using different parameters. Example
Take a look at the following example, with two functions that add numbers of a different kinds: Example
Instead of having two functions that can perform the same job it is better to overburden one. In the following example we will overload our Example
|
Notice: Multiple functions can be named the same as long as the parameters' number and/or types are distinct. |
C++ Recursion |
RecursionRecursion is a method of creating a function call itself. This method allows you to reduce complex problems into smaller problems that can be solved more easily. Recursion could be difficult to grasp. The best method to understand how it functions is to play around with it. Recursion ExampleThe process of adding two numbers together is easy However, adding a number of numbers is more challenging. In the below example, recursion is used to connect a number of numbers through breaking down to the simple job of adding 2 numbers Example
Example Explanation In the event that it is called, the
Since the function doesn't start calling itself when |
The programmer must be cautious when using recursion because it is quite easy to write an unfinished function that requires an excessive amount of processor or memory. However, when written correctly, recursion can be a very efficient and mathematically elegant approach to programming. |
For more information, Please visit Home
What's Your Reaction?
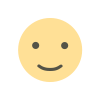
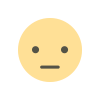
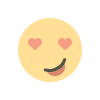
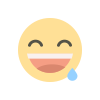
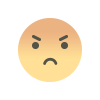
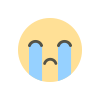
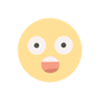