Unraveling the Magic of C++ Strings for Beginners: A Comprehensive Guide - w9school
Dive into the world of C++ strings with our beginner-friendly guide! Learn the basics, manipulation techniques, and unleash the power of strings effortlessly. Happy coding!
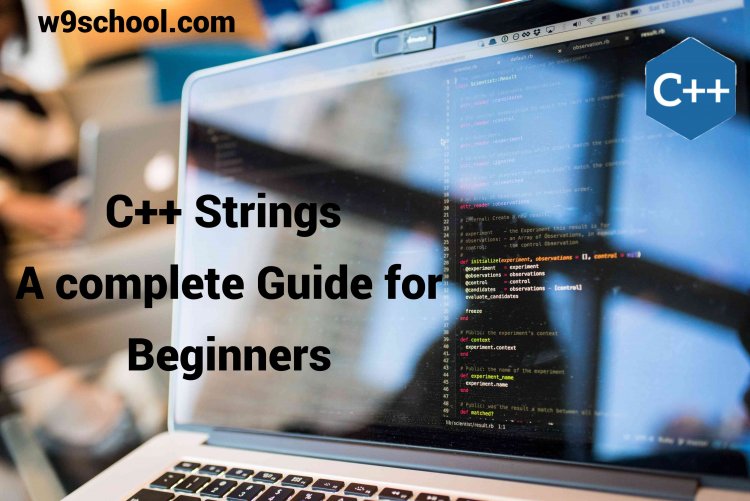
Welcome to the exciting world of C++ programming! If you're just getting started in programming, learning the fundamentals is vital. In this blog, we'll explore C++ strings - a foundational component used by many programs that enables manipulation and handling of textual data. Don't worry though; our straightforward explanation of this topic ensures even those without technical backgrounds can follow along easily! Understanding Strings in C++: Strings, in C++, are sequences of characters which could include letters, numbers, symbols or even spaces. You could think of strings as strings of letters strung together to form words or sentences which your program needs to interpret.C++ makes string manipulation simple with the string class from its Standard Template Library (STL). This class offers many functions and methods to make string manipulation straightforward. Unlike some other programming languages, C++ doesn't come equipped with its own string data type - instead relying on external services for string manipulation such as this string class from STL. |
C++ Strings |
Strings are used to store text. String Variables: A Example Create a variable kind
To make use of strings, you have to include a header file within the source code. This is the library: Example
|
String Concatenation |
It is possible to use the Example
In the above example we put a space before firstName to create an empty space between John Doe and John Doe in the output. You could, however, include a space using quotes ( Example
|
Append |
Strings within C++ is actually an object that contains functions that perform specific actions on strings. For instance, you can also combine strings with appsend(). Example
|
Adding Numbers and Strings |
WARNING! C++ uses the Strings are grouped together. |
If you multiply two numbers and the result is the following number: Example
If you are able to add two strings and the result is the string concatenation Example
If you attempt adding a number the string, you will encounter an error: Example
|
C++ String Length |
String Length To determine an estimate of the string's length utilize length(): Example
Tips: You might see certain C++ programs that utilize the Example
|
C++ Access Strings |
Access Strings You can find the characters contained in strings by using its index number in the square brackets This example shows only the the first line of myString: Example
NOTE:String indexes start with the number 0: [0] represents the initial character. "1" is the 2nd character, and so on. This example shows this example with the 2nd characters within myString: Example
Change String Characters To alter the meaning of a certain part of a string use the index number and then use single quotes: Example
|
C++ Special Characters |
Strings - Special Characters Since strings must be written in the quotes C++ will not understand the string and produce an error: Example
The best way to avoid this issue is to utilize to use the Backslash Escape character. Backslash (
The sequence \ Example
The sequence Example
The sequence "\\" Example
Other characters that are popular escape characters that are available in C++ are:
|
C++ User Input Strings |
User Input Strings There is a way to utilize extract operator Example
In reality, Example
In the previous example you'd expect that the program would print "John Doe", but it prints "John". Example
|
C++ String Namespace |
Omitting Namespace You may see C++ programs that can be run without the standard namespace library. The namespace line that uses the namespace is omitted and replaced by the std keyword which is followed by the operator for strings (and the cout) objects: Example
|
It's up to you whether you wish to use the namespace library that is standard or not. |
Test Yourself with C++ Exercises |
If you are ready to take test upon what what you've learnt then Click Here |
What's Your Reaction?
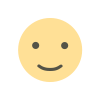
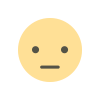
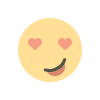
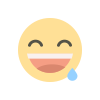
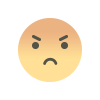
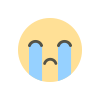
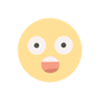