Python Inheritance Unveiled: Mastering Object-Oriented Evolution
Explore the pros and cons of Python inheritance. Uncover potential pitfalls and optimize your code with a comprehensive guide to object-oriented programming.
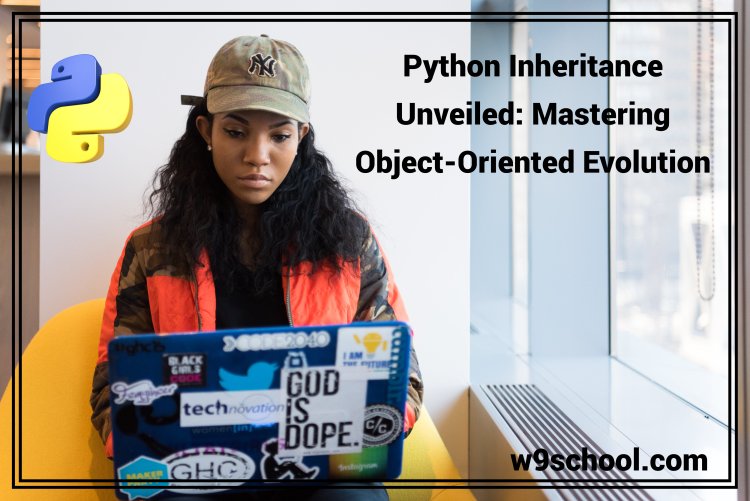
Python Inheritance |
Inheritance in PythonInheritance is one of the four pillars of object-oriented programming and an invaluable concept that can be applied across many scenarios. Understanding inheritance is critical for Python programmers as it enables code reusability and creates an easily understandable model structure, while also leading to lower development and maintenance costs. Types of ClassesInheritance is one of the core concepts in Python's object-oriented programming paradigm, enabling a child class (also called an inheriting class) to access methods and attributes from another class (known as its parent class) without duplicating all their methods or attributes themselves. This feature facilitates code reuse while improving program logic organization. Classes in Python are used to represent aspects of your computer program, such as data structures, behaviors, and relationships. They can be derived from other classes or created entirely. Class definitions use the def keyword; additionally, they can use docstrings for documentation. When creating a class that inherits from another, we refer to that parent class as its base or super class. A child class that inherits can then either override or customize its parent's methods and attributes as needed. Another form of inheritance in Python is composition, which involves creating one child class from multiple classes that implement its interface simultaneously.
Inheritance RelationshipsInheritance is a central concept in object-oriented programming. This feature enables classes known as child classes to inherit attributes and methods from another class known as their parent class; this process facilitates code reuse as well as the logical organization of code. Example: As a subclass of Cat, Dog inherits all its properties and methods, in addition to adding some unique ones of its own. To invoke methods from its parent class, child or derived classes must use the super() function. If no method can be found in their class, super() calls upon its method in its parent. Inheritance creates strong ties between classes, making it hard to modify their behaviors during run time. Composition allows looser coupling between them and makes changing them simpler. CompositionComposition is another method for code reuse that models relationships between classes. A composite class contains an instance of each component class and can leverage its implementation. Composition works well when you want only to utilize its interface without inheriting its implementation. An ideal example would be a hardware store which needs to represent all the tools it sells. All tools possess similar features but each tool also possesses specific attributes. To create this representation of all tools sold by them, a Base Class called Vehicle could be implemented, and subclasses for each type of tool could then add their specific attributes directly onto this base class. Composition is a great alternative to overriding base class methods with subclass methods; overriding them may become complex, unwieldy, and rigid in its implementation at run time. Multiple InheritancesThe object-oriented programming paradigm emphasizes inheritance as it allows you to reuse code. Through inheritance, a new class gains access to methods and attributes from existing classes; these classes are known as parent classes, while the ones giving access are known as child or subclass classes. Some languages allow multiple classes to act as the parent class of one child class; this practice is known as multilevel inheritance. Python supports multiple inheritances through its super() function, providing an easier way of calling methods on derived classes without specifying their base or parent class explicitly. When overridden methods exist in both classes, Python uses method resolution order to decide which class's method to call to avoid ambiguity and create clear results. |
Creating Classes |
Create a Parent ClassAny class could be a parent class thus the syntax is exactly the same as making any class you want to: Example Create a class titled
Create a Child ClassFor creating a class which inherits the functions of another class, you must send the class's parent as a parameter while designing the child class Example Create a class titled
|
NOTE: Use the |
The Student class now shares the same features and techniques similar to the Person class. Example Make use of the
|
Add the __init__() Function |
So far, we have created a child class which takes all the methods and properties of its parent. We would like to include the NOTE: The Example Include the
When you implement the Note: The child's To preserve the inheritance of that parent's Example
Today, we have added the function __init__() function and preserved the the parent class. Now we are now ready to add features to the |
Use the super() Function |
Python also includes an Example
When you use super() function, you can use the Add PropertiesExample Create an attribute called
In the following example, 2019, the date Example Include the
Add MethodsExample Include a procedure known as
If you create an operation in the child class using an identical name to a function within the parent class, the parent's inheritance method is overridden. |
Why Python inheritance? |
Inheritance is an essential concept in object-oriented programming, encouraging code reuse while at the same time encouraging bugs and unmaintainable code. One such issue is known as the diamond problem. This issue arises when two classes with similar methods inherit each other. Due to Python's name-mangling rules, methods beginning with two underscores ("__") become private, but they can still be accessed using their parent class's object. Inheritance is a fundamental concept in object-oriented programmingInheritance is a foundational concept in object-oriented programming, enabling new classes to inherit properties and methods from an existing class, increasing code reuse while helping you organize your codebase. Inheritance can be found across a range of real-world Python applications, including web frameworks and games. Inheritance can be implemented in different ways, from single to multiple inheritances. When inheriting methods and attributes from one class into another, this is known as single inheritance; when multiple inheritance is used instead, however, both classes become known as parent classes while their new descendants become subclasses of them all, creating a hierarchy known as polymorphism. Inheritance is a central concept in machine learning, and understanding it will help your coding skills to grow exponentially. It is particularly helpful when expanding the functionality of existing machine learning classes; for instance, you could use inheritance to create a class that extends the Random Forest classifier, Pandas dataframes, or any other machine learning tool. It promotes code reusabilityInheritance is a fundamental concept in Python's OOP paradigm and helps facilitate code reusability. It allows a class, known as the child class, to inherit attributes and methods from another class, known as its parent class, helping programmers organize code more logically while decreasing redundancies. Methods control class behavior, while attributes store information about objects. When creating a new class, its definition should include its parent class's name in parentheses at the end of its definition. For instance, class UndergraduateStudent(Student) indicates that it inherits the first and last attributes as well as the add_course_grade method from Student. You can use inheritance to build hierarchies of classes that share a common interface, but only when there is an explicit relationship between classes. Composition or aggregation might be better choices in situations in which no such relationship is present. It is a key aspect of object-oriented programmingInheritance is an integral component of object-oriented programming, as it allows one class to inherit attributes and methods from another (its "parent" class), improving code reuse as well as providing for better organization. Inheritance can be used in combination with other design patterns like aggregation and composition, although its application may be best when there is a clear relationship between classes. Furthermore, inheritance increases coupling between base classes and their derived ones so any change to one base class will impact all derived ones simultaneously. Single-level inheritance is the simplest form of inheritance, wherein there is only one parent class involved in inheritance. When used, the child class inherits all attributes and methods from its parent, with any necessary overrides occurring as necessary. Furthermore, using Python's super() function calls can also call any method belonging to its parent; multiple inheritance is supported too, allowing derived classes to inherit from multiple parents simultaneously and providing hierarchies of related classes. It is a fundamental concept in object-oriented programmingInheritance in Python is an impressive concept that allows classes to inherit attributes and methods from another class called its parent class, facilitating code reuse while keeping programs logically organized. However, inheritance isn't the only method available for reusing code; other approaches like composition and aggregation may prove more efficient in certain circumstances. Multiple inheritance can become very confusing when misused improperly, for example if multiple classes (A, B and C) inherit from class D with identical methods from him but inherit them in different versions: Class A gets one version while B gets another (known as the diamond problem). To prevent this from happening, leading underscores are an effective way of marking classes as abstract and abc can prevent objects of an abstract class from being created; although eager users still may create objects of AbstractEmployee class. |
Python Inheritance: Pros and Cons |
Inheritance in Python is a feature that allows a class (known as the child class) to inherit methods and attributes from another class (known as its parent class) for code reuse and better organization. One common use for inheritance in machine learning classes is to extend them with different functions that save both time and effort. This is an extremely helpful technique that can save both effort and time. ReusabilityInheritance is a powerful concept in OOPs that allows classes to inherit attributes and methods from another class (known as their parent). This provides code reusability and abstraction; however, excessive usage can make code unreadable and difficult to maintain. As well as encouraging reusability, inheritance promotes encapsulation, one of the core concepts in OOP. By decoupling complexity from its source classes to its descendants, inheritance makes code easier to read and maintain. Inheritance also facilitates polymorphism, which enables different classes to act similarly through a common interface. This makes the code more adaptable and flexible as the requirements of the program change. However, inheritance should only be used sparingly; other techniques like aggregation or composition may provide better solutions in situations with uncertain relationships between classes. ConvenienceInheritance allows developers to repurpose code efficiently. For instance, a base class that defines generic database operations can be inherited by different derived classes for different databases to reduce duplicate lines of code and improve code organization. But inheritance can also become problematic if overused; excessive inheritance results in complex class hierarchies that become difficult to navigate and maintain. Python inheritance allows developers to override methods in derived classes, providing developers with the flexibility to add functionality that differs from that implemented in base classes; this can be accomplished using super functions. Inheritance in Python can be an extremely powerful tool that enables developers to create modular and organized code structures. Furthermore, inheritance provides both reusability and extensibility benefits; however, it's essential that developers recognize when inheritance should be utilized versus considering alternatives like composition or aggregation as viable solutions. ScalabilityInheritance is an invaluable way of encouraging modularity and code organization. It enables developers to define a base class with common attributes and methods, then derive more specific classes from this base. In this way, inheritance provides developers with a powerful way of building flexible systems while decreasing duplication in code. At first glance, inheritance may appear to offer several disadvantages, especially when it comes to scaling. Multiple inheritances can create an inflexible hierarchy of classes that does not provide adequate flexibility or make modifications more complex due to references back to the parent class's methods. Multiple inheritance poses another problem for Python programming language users: there's no clear way of controlling how classes are resolved by Python, which can become particularly troublesome when classes in a hierarchy come from various third-party libraries. Composition provides a more flexible solution than hierarchical designs, as modifications can easily take place at runtime. ComplexityInheritance is a core concept in Python's object-oriented programming paradigm, enabling classes to share attributes and methods across classes for easier code organization and reuse across applications. By sharing attributes and methods between classes, inheritance allows developers to build modular and structured code structures while increasing code reusability through reused functionalities in multiple classes within applications. But inheritance can come with drawbacks. First off, multiple inheritance can make things very complex, and it can create confusing indirections that make code hard to read if its base class contains methods with similar names. Overall, inheritance should be used when its benefits outweigh its drawbacks. But it's important to remember that inheritance isn't the only way to reuse code; composition creates direct dependencies between classes for reuse purposes as well. Both methods allow reuse; however, inheritance tends to be preferable when its code can be passed along through multiple parents. |
For More informative blogs, Please visit Home
What's Your Reaction?
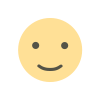
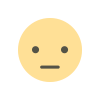
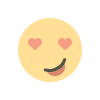
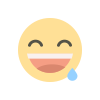
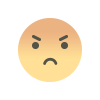
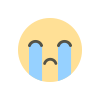
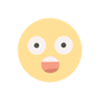