Unlocking the Potential of Python: A Guide to Learning with While Loops - w9school
Discover how Python's while loops can accelerate your learning journey in this comprehensive guide to Python programming.
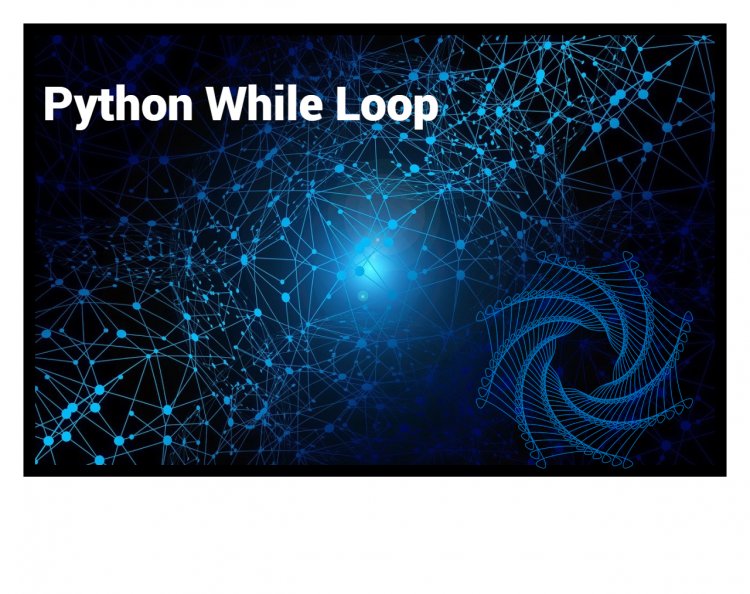
Python Loops |
Python has a pair of fundamental loop commands:
|
The While Loop |
A 'while' loop is a control structure in Python that allows you to execute a block of code continuously as long as a given condition remains true. This loop is used when you want to perform an action multiple times, and the number of iterations is not known beforehand. The loop will continue running until the specified condition becomes false. Certainly, the |
Uses of Python While Loop |
The
Remember that while " |
Python While Loop Syntax |
Here's the syntax for a '
In this syntax:
Here's a simple example that utilizes a while loop to print integers from 1 to 5:
In this case, the loop continues to execute as long as count is less than or equal to 5. It prints the value of Remember that it's important to ensure that the condition in the ' The while loop requires relevant variables to be ready, in this example, we need to define an indexing variable, count, which we set to 1. |
The break Statement |
The In the context of a ' Here's an example of how the
In this example, the loop condition is ' This usage of It's important to use the |
The continue Statement |
The The Here's a simple example using a '
In this example, the 'continue' statement is encountered when the value of 'num' is even, and the code below it for the current iteration is skipped. As a result, only odd numbers are printed. Here's another example using a
In this example, the ' The |
The else Statement |
In Python, the ' Here is an example to illustrate the use of the
In this example, the The ' It's important to note that the ' |
Test Yourself With Exercise |
What's Your Reaction?
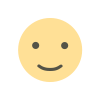
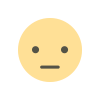
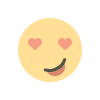
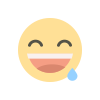
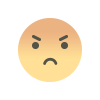
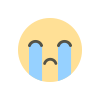
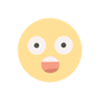